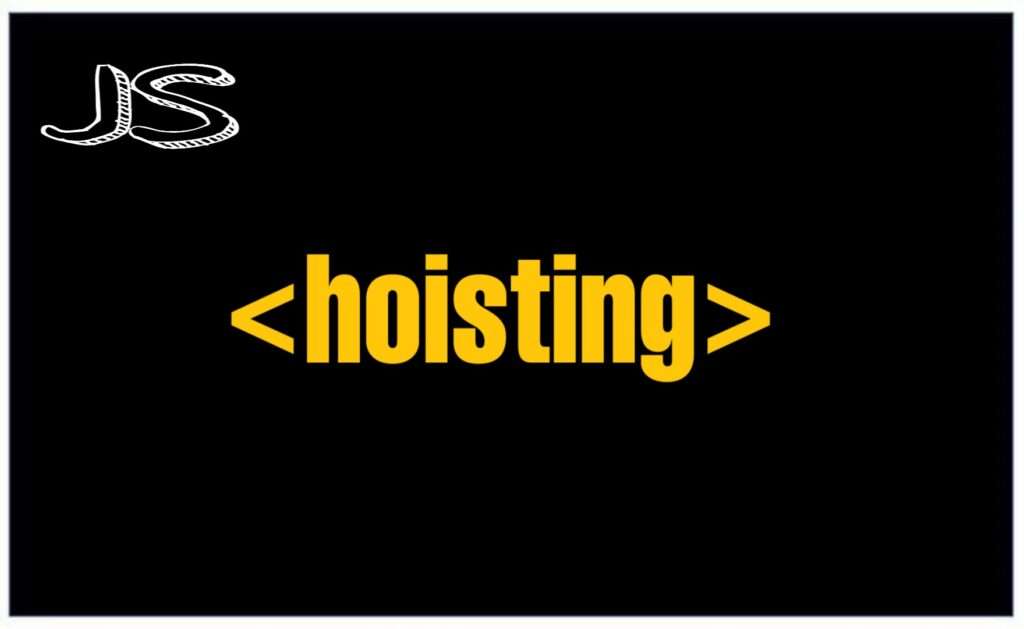
Hoisting in JavaScript Most of the language like c, c++ you have to first declare the variable before make use of it but this is not the case in JavaScript you can use the variable before declaring it even functions. Here the word hoisting exactly means that the JavaScript compiler will take the var variable to the top and initialized it with undefined, no matter what you initialized in it at declaration time.
There are two phases of execution in javascript engine when an execution context is created
1) Declaration
2) Execution
In first phase of execution all the declared variables and functions are stored in memory and at the time of storing them in memory variables are assigned to undefined.
And in second phase i. e. execution phase actual values are assigned to that memory locations
And in simple words declaration phase of that execution context is called as hoisting . Hoisting is a concept in JavaScript, not a feature.
a = 3;
console.log(a);. // prints 3
var a;
b = 5;
console.log(b); // error
let b;
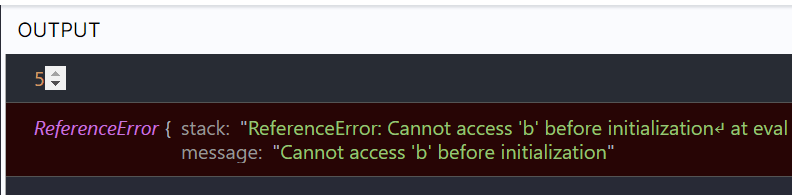
Hoisting in Functions: Hoisting in JavaScript
alert(add(3, 3)); // 6
function add(num1, num2)
{
return num1 + num2;
}
Output:
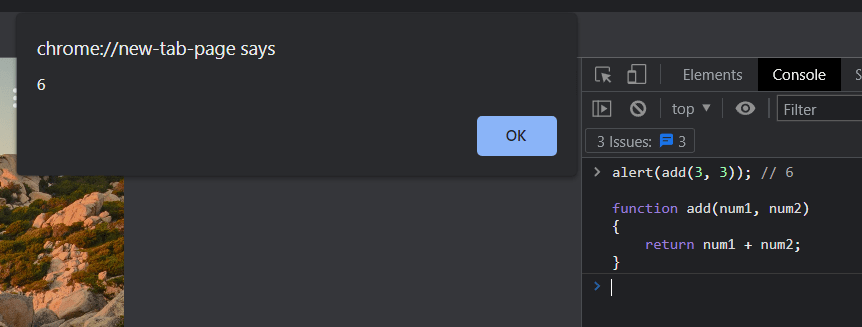

- JavaScript compiler moves variables and function declaration to the top and this is called hoisting.
- Hoisting is a concept in JavaScript, not a feature.
Hoisting in JavaScript