What is component ?
Components are the most basic UI building block of an Angular app. An Angular app contains a tree of Angular components. A component includes a TypeScript class with a @Component()
decorator, an HTML template, and styles. The @Component()
decorator specifies the following Angular-specific information.
Let’s understand components in a better way, consider that we are building a page for an application. The features in the page include the home, header, footer and navigation and content area and many more sections. Instead of building a single page with all these features, we can choose to split the page into components, which help us to understand easy way as well as we can manage our application easily.
In the above example, we can say that the home, header, footer, content area, navigation and so on are separate components of the page, but when the user views it on the website through any device, it will show as a single page.
- Components are used to break up the application into smaller components.
- We are using @Component meta-data for creating component and import in the ngModule for including in the application.
- Components are used to create UI widgets.
- Only one component can be present per DOM element.
- @View decorator or templateurl/template are mandatory.
ng g c <component_name>
OR
ng generate component <component_name>
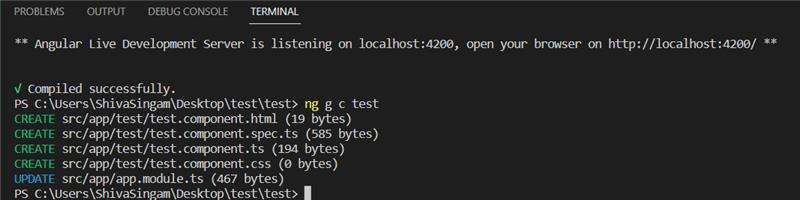
We have some files in each and every component. The first file is
test.component.html:
<p>test works!<p>
<h1>Fullstackadda.com</h1>
test.component.css:
h1{
color: green;
font-size: 30px;
}
test.component.ts:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-test',
templateUrl: './test.component.html',
styleUrls: ['./test.component.css']
})
export class DemoComponent{
message = 'Hello, World!';
}
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-test',
templateUrl: './test.component.html',
styleUrls: ['./test.component.css']
})
export class DemoComponent{
message = 'Hello, World!';
}
app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
import { TestComponent } from './test/test.component';
@NgModule({
imports: [ BrowserModule ],
declarations: [ AppComponent, TestComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
Output:
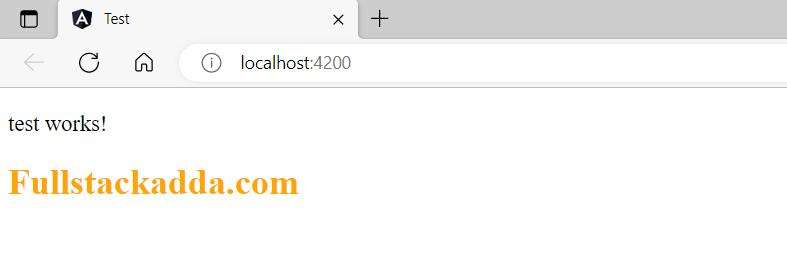