In javascript, var, let and const are ways to create variables. They all behave differently:
let and const are introduced in ES6 Edition:
Using var:
var demo = "shiva";
console.log(demo)
Using let:
let demo = "shiva";
console.log(demo)
Using const:
let demo = "shiva";
console.log(demo)
we will look at the differences between these keywords. var
The main differences are scope, hoisting, and reassignment.
Scope
When using var
, the scope depends on whether you declare it inside or outside a function. If declared outside a function, its scope is global – meaning it can be used anywhere. Inside a function, its scope is limited to that function.
For let
and const
, there is block scoping. With block scoping, the scope is limited to the block (defined with curly braces: {}
) that the variable is declared in. So for instance, if you have a function that contains an if(…) {…}
statement, and inside that statement you declare a let
, that let
is not available to the rest of the function. If you declare it as a var
instead, then it will be available to the rest of the function after all.
var | let | const |
---|---|---|
Variables declared with var are in the function scope. | Variables declared as let are in the block scope. | Variables declared as const are in the block scope. |
Hoisting
With var
, variables will be hoisted to the top. You can attempt to access them before they are initialized, and while their values will be undefined, you won’t receive an error, when it would actually be better if you did. With let
and var
, you’ll receive a ReferenceError, which is preferable.
var | let | const |
---|---|---|
Allowed | Not allowed | Not allowed |
name = "Avatar"; //using var keyword
console.log(name);
var name;
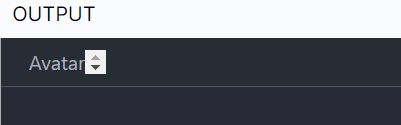
name = "Avatar"; //using let keyword
console.log(name);
let name;
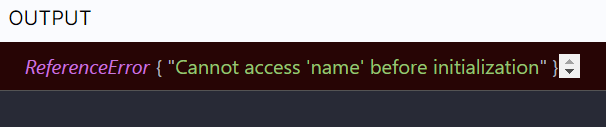
name = "Avatar"; //using const keyword
console.log(name);
const name;
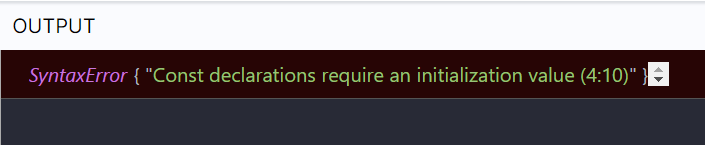
Reassignment
With both var
and let
, reassignment is possible. With const, reassignment like that is impossible.
var | let | const |
---|---|---|
Allowed | Allowed | Not allowed |
Where to use ?
- Use
const
everywhere. As long as the value is not supposed to change,const
is preferred. - Anywhere that a variable’s value can change, use
let
. - Don’t use
var
. Ever. At all. You don’t need it anymore.