A conditional statement is a statement in which we check for some conditions , if the condition is true we execute some block of code and if the condition evaluate to false we execute some other block of code.
In JavaScript, conditional statements guide actions based on a specific true-or-false logic.
There are four approaches to JavaScript conditional statements, which namely are:
- if
- if/else
- else if
- Switch
if: This conditional specifies a condition which triggers an action if found to be true.
if…else: This conditional specifies an action to be triggered if a certain condition is true and an alternative action to be triggered if the condition is false.
else if: This conditional statement specifies a series of conditions that trigger an action, and an alternative script is executed if all specified conditions are false.
switch: The switch condition is a way of executing different scripts based on the set conditions.
if Statement:
if (condition) {
// if the condition if the condition in true than block of code to be executed
}
Example:
const age = 20;
if (age > 18) {
alert("You can vote");
}
Output:
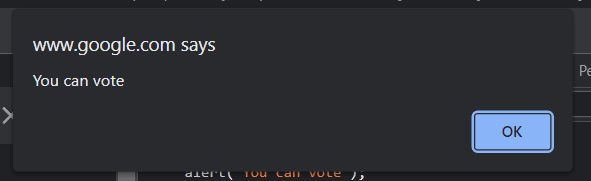
else Statement:
if (condition) {
// block of code to be executed if the condition is true
} else {
// block of code to be executed if the condition is false
}
const age = 16;
if (age > 18) {
alert("You can vote");
}else{
alert("You cannot Vote");
}
Output:
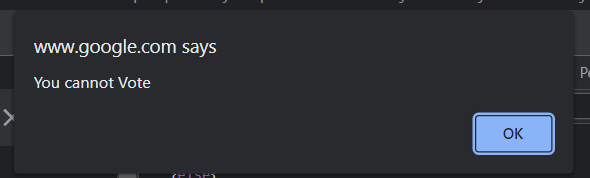
else if Statement:
if (conditions) {
statements;
} else if (conditions) {
statements;
} else {
statements;
}
const age = 20
if (age >= 21) {
alert("You can vote and drink!");
} else if (age >= 18) {
alert("You can vote, but can't drink.");
} else {
alert("You cannot vote or drink.");
}
Click here to learn about Switch Case Statement

- if: This conditional specifies a condition which triggers an action if found to be true.
- if…else: This conditional specifies an action to be triggered if a certain condition is true and an alternative action to be triggered if the condition is false.
- else if: This conditional statement specifies a series of conditions that trigger an action, and an alternative script is executed if all specified conditions are false.