Till now we wrote many programs, we have designed our program around functions i.e. blocks of statements which manipulate data. This is called the procedure-oriented way of programming.
There is another way of organizing your program which is to combine data and functionality and wrap it inside something called an object. This is called the object oriented programming paradigm.
Most of the time you can use procedural programming, but when writing large programs or have a problem that is better suited to this method, you can use object oriented programming techniques.
In this tutorial we will learn about object oriented programming.
Main concepts in OOP’s
- Class
- Objects
- Polymorphism
- Encapsulation
- Inheritance
- Abstraction
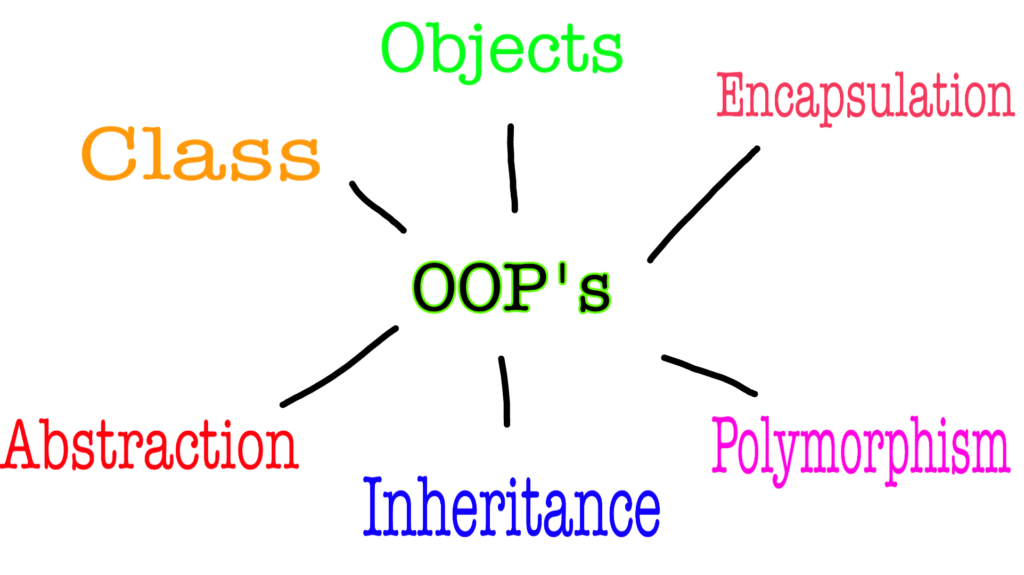
Class
The class is a user-defined data structure that binds the data members and methods into a single unit. Class is a blueprint or code template for object creation. Using a class, you can create as many objects as you want.
Create a Class in Python
In Python class
keyword is used to create a class.
class class_name:
<statement 1>
<statement 2>
.
.
<statement N>
class_name
: name of the classstatements
: Attributes and methods.
Example:
In this example we are creating a Employee class with name id number and role are the instance variables.
class Employee:
def __init__(self, name, idNum, role):
#instance variables
self.name = name
self.idNum = idNum
self.role = role
#instance methods
def show(self):
print('Name:', self.name, 'IdNum:', self.idNum, 'role:', self.role)
def work(self):
print(self.name, 'working as a', self.role)
What is _init_ method ?
__init__ is a reserved method in python classes. It is known as a constructor in object oriented concepts. This method called when an object is created from the class and it allow the class to initialize the attributes of a class
Objects
An object is built from a class, and is the thing, which holds the actual data, and has the actual behaviors. An object is created using the class name. When we create an object of the class, it is called instantiation. The object is also called the instance of a class. It may be any real-world object like a chair, table, pen, etc. Integers, strings, floating-point numbers, even arrays, and dictionaries, are all objects.
Creating an Object
obj = Employee()
Example:
class Employee:
def __init__(self, name, idNum, role):
#instance variables
self.name = name
self.idNum = idNum
self.role = role
#instance methods
def show(self):
print('Name:', self.name, 'IdNum:', self.idNum, 'role:', self.role)
def work(self):
print(self.name, 'working as a', self.role)
display = Employee('Surya', 'SG1280', 'Software Engineer')
# call methods
display.show()
display.work()
Name: Surya IdNum: SG1280 role: Software Engineer
Surya working as a Software Engineer
Polymorphism
Polymorphism is a concept in OOP which is more or less language independent.
Polymorphism is the concept of an object having multiple forms; it is often implemented via multiple inheritance, but doesn’t have to be.
Normally in OOP, the ‘form’ of an object is defined by the attributes and methods which are available, so in Python polymorphism can be implemented simply by an object have more than one well defined interface available.
A good example is a Python dictionary :
- It can act as a Boolean (empty dictionaries are treated as False)
- It can act as an Iterable (it can be used in for loop)
- it acts as a Mapping (it associated keys with values)
Consider a simple example of polymorphism
def add(a, b, c = 0):
return a + b + c
print (add(2, 5))
print (add(2, 5, 25))
7
32
Polymorphism in classes
We can perform the polymorphism in classes, let’s consider an example
class Car():
def speed(self):
print("Max speed is 120KPH")
def millage(self):
print("30 KM Millage per Liter")
class Bike():
def speed(self):
print("Max speed is 90KPH")
def millage(self):
print("50 KM Millage per Liter")
obj_1 = Car()
obj_2 = Bike()
for vechicle in (obj_1, obj_2):
vechicle.speed()
vechicle.millage()
Max speed is 120KPH
30 KM Millage per Liter
Max speed is 90KPH
50 KM Millage per Liter
Polymorphism with Function and Objects
We can perform the polymorphism in functions and objects also, let’s consider an example
class Car():
def speed(self):
print("Max speed is 120KPH")
def millage(self):
print("30 KM Millage per Liter")
class Bike():
def speed(self):
print("Max speed is 90KPH")
def millage(self):
print("50 KM Millage per Liter")
# normal function
def vechicle_details(obj):
obj.speed()
obj.millage()
car = Car()
bike = Bike()
vechicle_details(car)
vechicle_details(bike)
Max speed is 120KPH
30 KM Millage per Liter
Max speed is 90KPH
50 KM Millage per Liter
Encapsulation
Encapsulation is a process of binding the data and methods in a single unit.
For example, when you create a class, it means you are implementing encapsulation. A class is an example of encapsulation as it binds all the data members (instance variables) and methods into a single unit.
class Employee:
def __init__(self, name, salary, role):
self.name = name
self.salary = salary
self.role = role
# method
def show(self):
print("Name: ", self.name, 'Salary:', self.salary)
# method
def work(self):
print(self.name, 'is working as', self.role)
# creating object of a class
emp = Employee('Surya', 50000, 'Software Dev')
emp.show()
emp.work()
Name: Surya Salary: 50000
Surya is working as Software Dev
Encapsulation is a way to can restrict access to methods and variables from outside of class. Whenever we are working with the class and dealing with sensitive data, providing access to all variables used within the class is not a good choice.
Inheritance
Inheritance is a mechanism in object-oriented programming (OOP) that allows a new class to be defined by inheriting properties and methods from an existing class. The existing class is called the base class or the parent class, and the new class is called the derived class or the child class.
Inheritance enables code reuse and promotes the DRY (Don’t Repeat Yourself) principle in programming. By inheriting from a base class, a derived class can inherit the attributes and behaviors of the base class, and can also add new attributes and behaviors of its own.
In python, you can use the class DerivedClassName(BaseClassName)
syntax to create a derived class that inherits from a base class. The base class is specified in the parentheses after the derived class name.
Inheritance is the procedure in which one class inherits the attributes and methods of another class. The class whose properties and methods are inherited is known as the Parent class. And the class that inherits the properties from the parent class is the Child class.
class parent_class:
body of parent class
class child_class(parent_class):
body of child class
class Person(object):
#constructor
def __init__(self, name, idNum):
self.name = name
self.idNum = idNum
def display(self):
print(self.name)
print(self.idNum)
def details(self):
print("My name is {}".format(self.name))
print("IdNumber: {}".format(self.idNum))
# child class
class Employee(Person):
def __init__(self, name, idNum, salary):
self.salary = salary
Person.__init__(self, name, idNum)
def details(self):
print("My name is {}".format(self.name))
print("IdNumber: {}".format(self.idNum))
show = Employee('Surya', 'SG1280', 50000)
# calling a function of the class Person using
# its instance
show.display()
show.details()
Surya
SG1280
My name is Surya
IdNumber: SG1280

- __init__ is a reserved method in python classes. It is known as a constructor in object oriented concepts.
- Class is a blueprint or code template for object creation.
- An object is built from a class, and is the thing, which holds the actual data, and has the actual behaviors.
- Polymorphism is the concept of an object having multiple forms; it is often implemented via multiple inheritance, but doesn’t have to be.
- Encapsulation is a process of binding the data and methods in a single unit.