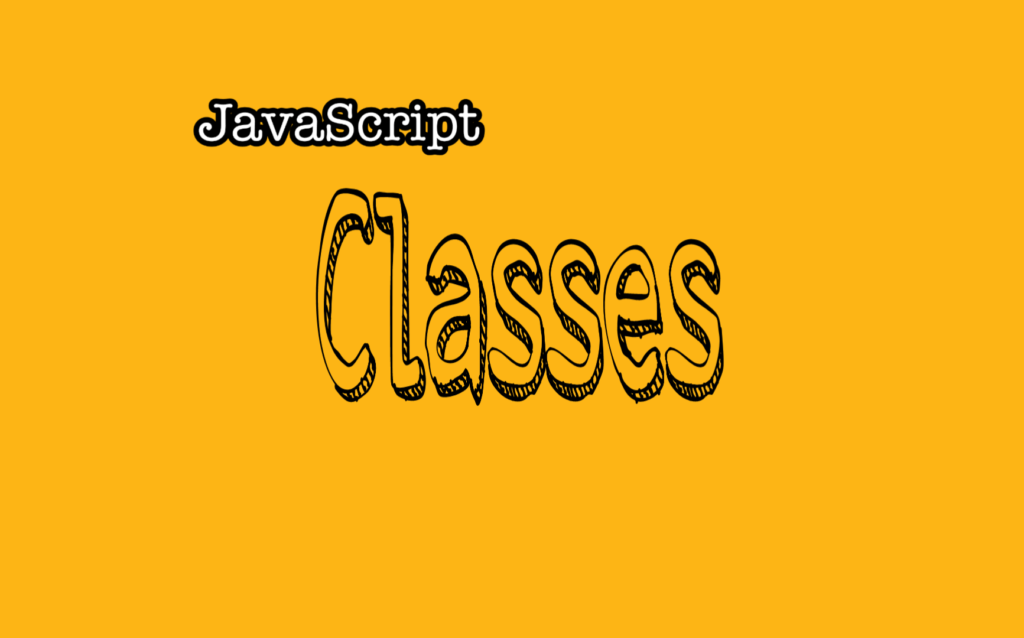
JavaScript Classes are introduced in ES6(ECMAScript 2015).
Since everything in javaScript is an object, so an instance of a class is an object. A class in javascript is basically a blueprint or template of the object. It encapsulates data and functions that manipulate data, grouping data and functionality together. JavaScript classes are considered syntactic sugar.(“syntactic sugar” as it makes things easier to read, i.e. “sweeter” for human use. In this case, for developers who prefer object-oriented programming.)
“class” keyword is used to create a class in javascript
class ClassName {
constructor() { ... }
}
Example:
class person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
const person1 = new person("Vasu", "28");
console.log(person1.name);
console.log(person1.age);
Vasu
28
Example 2:
class Person {
constructor(name) {
this.name = name;
}
sayName() {
console.log(this.name);
}
}
let test = new Person('Surya Singam');
test.sayName();
Surya Singam
What is constructor ?
A Constructor is a block of code similar to a method that’s called when an instance of an object is created.
- A constructor doesn’t have a return type.
- Unlike methods, constructors are not considered members of a class.
- A constructor is called automatically when a new instance of an object is created.
class Person {
constructor(name) {
this.name = name;
}
getName() {
return this.name;
}
}