What is Anonymous Functions ?
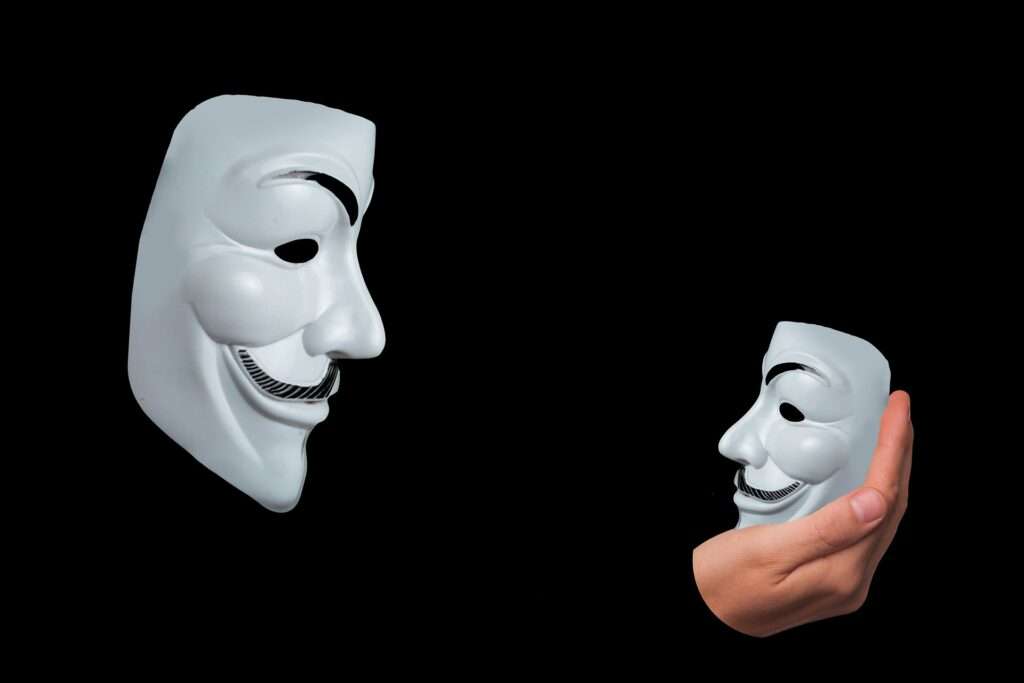
An anonymous functions is a function that was declared without any named identifier to refer to it. As such, an anonymous function is usually not accessible after its initial creation.
Example:
Named function:
function hello() {
alert('Hello world');
}
hello();
Anonymous function:
In below example, we define an anonymous function that prints a message(“I am anonymous”) to the console. The function is then stored in the txt variable. We can call the function by invoking txt().
var txt = function() {
alert('I am anonymous');
}
txt();
Example 2: Arguments to the anonymous function.
var msg = function (name) {
console.log("Hello ", name);
};
msg("RajiniKanth");
Hello RajiniKanth
Use of Anonymous Functions ?
- Anonymous functions are useful to avoid name collisions and this applies to all languages that support it.
- JavaScript is a High Order language, which makes it able to pass functions as an argument to other functions. Previously we used to pass the name of the function like this.
function timeOut(){
console.log(“i will console after 1 sec”)
}
setTimeout(myFunction(), 1000)
As the Anonymous function does not have a name, we can pass directly as the arguments to other functions. We do not need explicitly declare the function outside the argument. Here it goes like
setTimeout(timeOut() {
conole.log(“i will console after 1 sec”)
}, 1000)

- Anonymous Function is a function that does not have any name associated with it.
- An anonymous function is not accessible after its initial creation, it can only be accessed by a variable it is stored in as a function as a value.
- An anonymous function can also have multiple arguments, but only one expression.