Arrow functions is one of the features introduced in the ES6 version of JavaScript. It allows you to create functions in a cleaner way compared to regular functions.
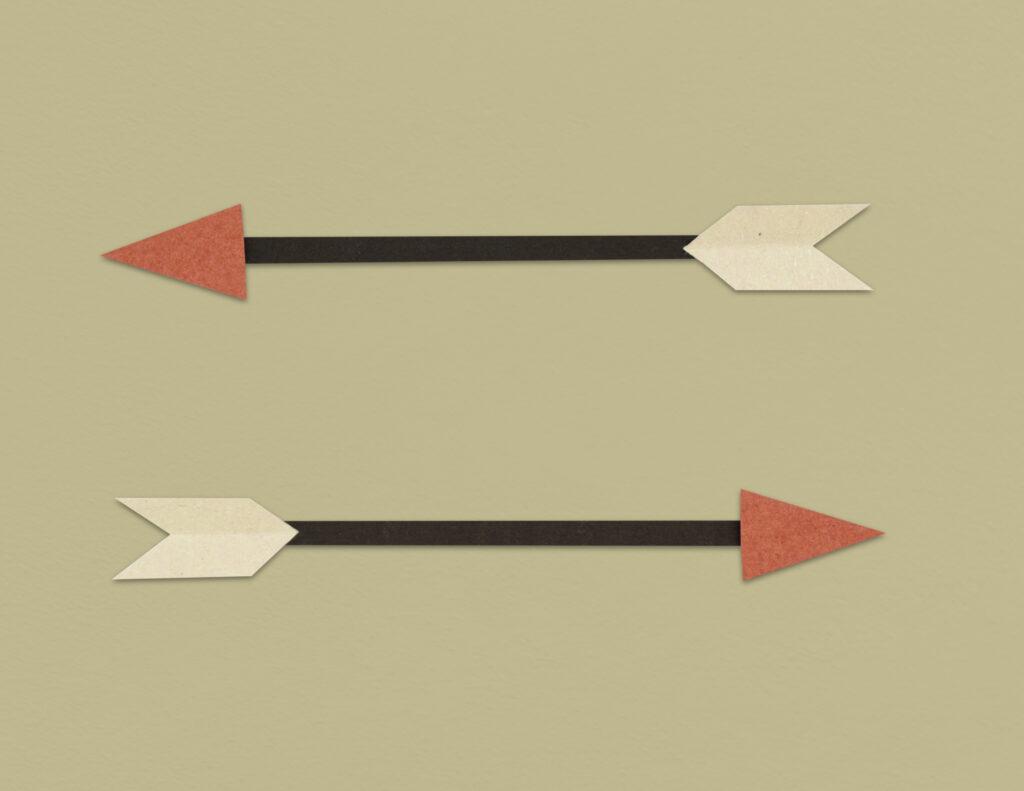
Arrow function provide lexical binding, meaning you get the control back on the value of this inside the function’s body. By getting the control back, I mean that it’s fairly easy to know which object this refers to just by looking at the code where your arrow function is defined (and not called).
Normal Function :
JavaScriptmsg = function() { return "Hello World!"; }
Arrow Function :
JavaScriptmsg = () => { return "Hello World!"; }
Instead of Writing
JavaScriptfunction add(n1, n2) { return n1 + n2 } // You can write const add = (n1, n2) => n1 + n2
You can remove the brackets and the return
keyword if function has only one statement, and the statement returns a value.
—> Arrow functions take the this from their surroundings (called lexical binding).
JavaScript// ES5 way var result = { name: "Hello", data: function data() { setTimeout(function() { console.log(this.name); }.bind(this), 5000); } }; // ES6 way with arrow functions var result = { name: "Hello", data: function data() { setTimeout(() => { console.log(this.name); }, 5000); } };
This syntax allows an implicit return when there is no body block, resulting in shorter and simpler code.
More Examples:
JavaScriptlet numbers = [1,2,3,4]; let test1 = numbers.map((item) => item * 5); let test2 = numbers.filter((item) => item < 3); let test3 = numbers.reduce((result, item) => result + item); console.log(test1); console.log(test2); console.log(test3);
Output[5, 10, 15, 20] [1,2] 10
When to use Arrow Functions ?
You should use arrow function when this is required to be bound to the context, and not function itself.
You can use them with methods such as map and reduce which makes the code more readable.
Here’s a table comparing the differences between normal functions and arrow function in JavaScript:
Feature | Normal Function | Arrow Function |
---|---|---|
Syntax | Uses the function keyword followed by the function name and parameter list | Uses a parameter list followed by the => operator and the function body |
Implicit return | Does not have an implicit return statement | Has an implicit return statement if the function body is a single expression |
this binding | Has its own this value, which can change depending on how it is called | Inherits the this value from the enclosing lexical scope |
Arguments object | Has its own arguments object that contains all the arguments passed to the function | Does not have its own arguments object |
Method syntax | Can be used as a method on an object using the object’s context for this | Can be used as a method on an object, but does not have its own this value |
Function hoisting | Can be hoisted to the top of the current scope, allowing them to be called before they are declared | Can’t be hoisted, so they must be declared before they are used |
Function length | The length property returns the number of named arguments defined in the function | The length property returns the number of parameters defined in the function |
Advantages of Arrow Functions:
- Shorter syntax: Arrow function provide a shorter and more concise syntax for defining functions, by using the => operator instead of the function keyword. This can help make your code more readable and reduce visual clutter.
- Implicit return: Arrow function have an implicit return statement, which means you don‘t need to use the return keyword if the function has a single expression. This can make your code more concise and easier to read.
- Lexical this binding: Arrow function have a lexical this binding, which means they inherit the this value from the enclosing function. This can help avoid common errors and make your code more predictable.
- Compatibility with functional programming: Arrow functions are compatible with functional programming concepts such as higher-order functions, map, filter, and reduce, which can help make your code more functional and easier to reason about.
- Faster performance: Arrow function are generally faster than traditional functions, because they have a simpler syntax and a more efficient implementation. This can help improve the performance of your code, especially in performance-critical applications.