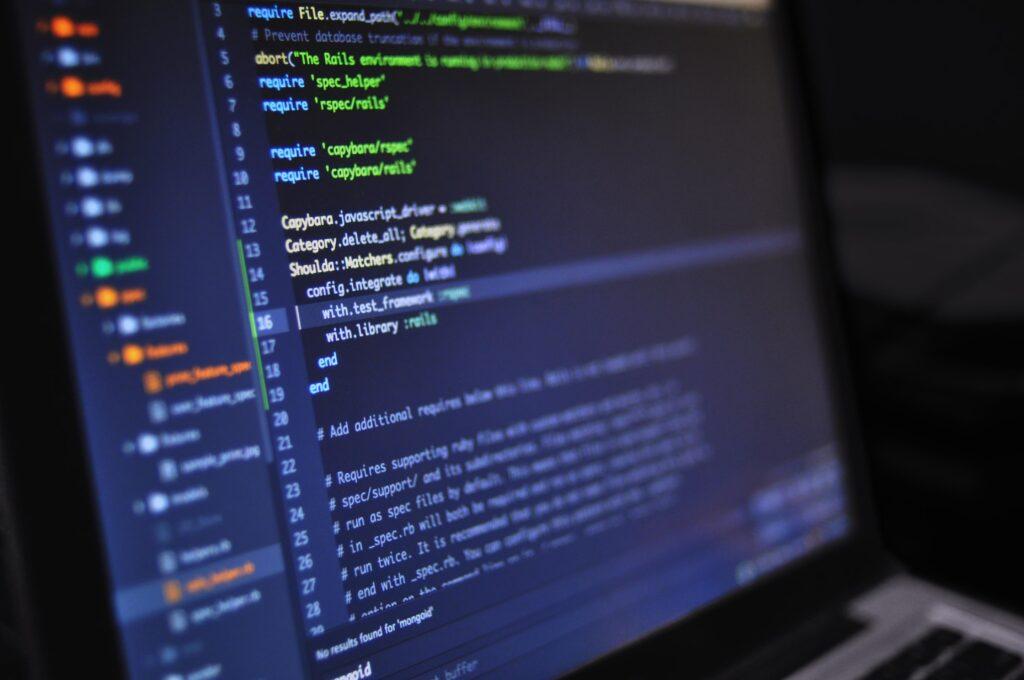
Angular forms are a way to handle user input in an Angular application. They provide a way to create and validate forms, as well as handle errors and data binding. Angular provides several different types of forms, including template-driven forms and reactive forms. They are used to create the form and form elements, and to handle validation and data binding.
Here is an example of a template-driven form in Angular:
<form #form="ngForm" (ngSubmit)="submitForm(form)">
<label>
Name:
<input type="text" name="name" ngModel required>
</label>
<br>
<label>
Email:
<input type="email" name="email" ngModel required>
</label>
<br>
<button type="submit" [disabled]="form.invalid">Submit</button>
</form>
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
})
export class ExampleComponent {
submitForm(form) {
console.log(form.value);
}
}
This example creates a simple form with two input fields for name and email, and a submit button. The #form
template reference variable is used to access the form’s properties and methods, such as the invalid
property to check if the form is valid. The ngModel
directive is used to bind the input fields to the component’s data. The required
attribute is used for validation.
On the other hand, here is an example of a reactive form in Angular:
import { Component } from '@angular/core';
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
@Component({
selector: 'app-example',
template: `
<form [formGroup]="form" (ngSubmit)="submitForm()">
<label>
Name:
<input type="text" formControlName="name" required>
</label>
<br>
<label>
Email:
<input type="email" formControlName="email" required>
</label>
<br>
<button type="submit" [disabled]="form.invalid">Submit</button>
</form>
`,
})
export class ExampleComponent {
form: FormGroup;
constructor(private fb: FormBuilder) {
this.form = this.fb.group({
name: ['', Validators.required],
email: ['', [Validators.required, Validators.email]],
});
}
submitForm() {
console.log(this.form.value);
}
}
This example creates a form group and form controls using the FormBuilder. The form controls’ values and validation status are bound to the form group. The formControlName
directive is used to bind the input fields to the form controls. The required
and email
validators are used for validation. Angular Forms
Both examples will log the form’s value (i.e the values entered by the user) when the form is submitted.
Advantages of Forms :
- Two-way data binding: Angular forms provide two-way data binding, which means that changes made to the form data are immediately reflected in the user interface and vice versa. This eliminates the need for manual updates to the form and reduces the likelihood of errors.
- Validation: Angular forms provide built-in validation support that allows you to validate user input and display error messages when the input is invalid. This makes it easy to create forms that enforce data integrity and prevent users from submitting incorrect or incomplete data.
- Dynamic forms: Angular forms support dynamic forms, which means that you can add and remove form fields dynamically based on user input. This allows you to create forms that adapt to the user’s needs and provide a more personalized experience.
- Form controls: Angular forms provide a rich set of form controls that can be easily customized to fit your specific needs. These controls include input fields, checkboxes, radio buttons, select boxes, and more.
- Reactive forms: Angular forms also provide reactive forms, which are a powerful and flexible way to manage form data. Reactive forms use observables to manage form data, making it easy to react to changes in the form and perform advanced form processing.
- Better user experience: Angular forms allow you to create forms that provide a better user experience. For example, you can use form validation to prevent users from submitting invalid data, which can save them time and frustration. You can also use dynamic forms to create more personalized forms that adapt to the user’s needs.
- Testing: Angular forms are designed with testing in mind, which makes it easy to test your forms and ensure that they are working as expected. You can use automated tests to verify that your forms are validating user input correctly, handling errors gracefully, and providing a smooth user experience.
- Customization: Angular forms provide a high degree of customization, which allows you to create forms that match your application’s specific needs. You can customize form controls, add custom validators, and use custom error messages to create forms that provide a seamless user experience and meet your application’s unique requirements.