CSS can be used to style HTML forms to make them look more visually appealing and user-friendly. CSS Forms
Here are a few examples of how CSS can be used to style forms:
- Changing the color and font of form labels:
label {
color: blue;
font-size: 14px;
font-family: Arial, sans-serif;
}
- Changing the background color of input fields when they are focused:
input:focus {
background-color: #f5f5f5;
}
- Adding a border and padding to input fields:
input {
border: 1px solid #ccc;
padding: 10px;
}
- Changing the color and font of the submit button:
input[type="submit"] {
background-color: blue;
color: white;
font-size: 16px;
}
- Making the form responsive by adjusting the layout for different screen sizes
@media only screen and (max-width: 600px) {
form {
width: 80%;
}
input {
width: 100%;
}
}
It is also possible to create customized form controls using CSS, and these can be made to look like traditional controls using checkbox, radio, select etc.
To create a form, you will need to do the following:
- Begin by creating an HTML document. This can be done by creating a new file in your text editor and saving it with an
.html
file extension. - Inside the
<body>
of your HTML document, add the<form>
element. This element will serve as a container for all of the form elements that you will add to your form. - Inside the
<form>
element, you can add various form elements such as<input>
,<textarea>
, and<select>
. These elements allow you to collect information from the user, such as their name, email address, and comments. - To make the form functional, you will need to add a submit button. This can be done using the
<button>
element.
For more about Forms ClickHere
Example: CSS Forms
<!DOCTYPE html>
<html>
<head> </head>
<body>
<form>
<label for="name">Name:</label><br>
<input type="text" id="name" name="name"><br>
<label for="email">Email:</label><br>
<input type="text" id="email" name="email"><br>
<label for="comments">Comments:</label><br>
<textarea id="comments" name="comments"></textarea><br><br>
<button type="submit">Submit</button>
</form>
</body>
</html>
Output:
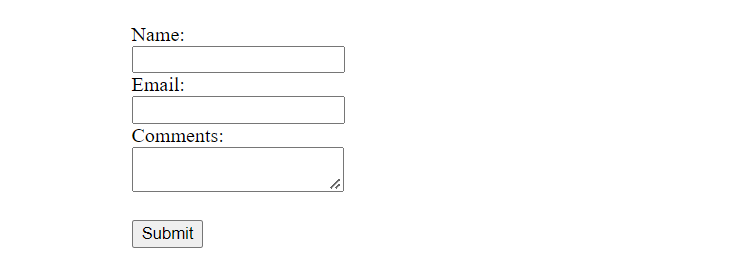
Now, we can style those forms using CSS
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style>
body {
font-family: sans-serif;
}
input[type=text],
select,
textarea {
width: 100%;
padding: 12px;
border: 1px solid #ccc;
border-radius: 4px;
box-sizing: border-box;
margin-top: 6px;
margin-bottom: 16px;
resize: vertical;
}
input[type=submit] {
background-color: grey;
color: white;
padding: 12px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
}
input[type=submit]:hover {
background-color: blue;
}
.container {
border-radius: 5px;
background-color: #f2f2f2;
padding: 20px;
}
</style>
</head>
<body>
<h3>Example of Form</h3>
<div class="container">
<form>
<label for="firstname">First Name</label>
<input type="text" id="firstname" name="firstname" placeholder="Your name..">
<label for="lastname">Last Name</label>
<input type="text" id="lastname" name="lastname" placeholder="Your last name..">
<label for="country">Country</label>
<select id="country" name="country">
<option value="India">India</option>
<option value="canada">Canada</option>
<option value="usa">USA</option>
</select>
<input type="submit" value="Submit">
</form>
</div>
</body>
</html>
Output:
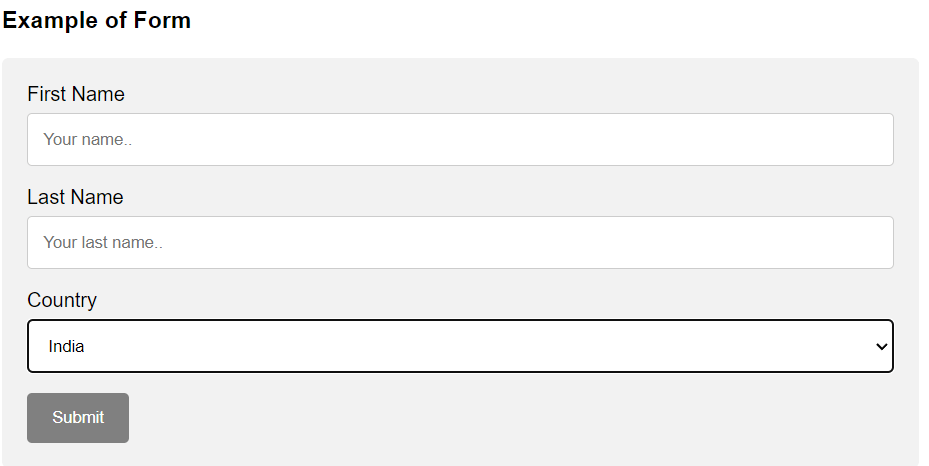

- Form tag is used for creating a forms in HTML.
- Inside the
<form>
element, you can add various form elements such as<input>
,<textarea>
, and<select>
. These elements allow you to collect information from the user, such as their name, email address, and comments. - CSS can be used to style HTML forms to make them look more visually appealing and user-friendly.