What is a functions ?
Functions are the block of code designed statements that perform a particular task. Functions in JavaScript
A function is a group of code that is reusable, it helps you to organize your program, make your code less polluted and helps you make use of the same code that you want to use again and again.
Function starts with the keyword function, followed by the name of the function you want to create in JavaScript.
Syntax:
The basic syntax for declaring a function are mention below:
function functionName() {
// Code to be executed
}
With parameters
function name(parameter1, parameter2) {
// code to be executed
}
Another example of
// Creating a function
function alertBox() {
alert("Hello welcome to Fullstackadda.com");
}
// Calling function
sayHello(); // it give the alert msg
Output:
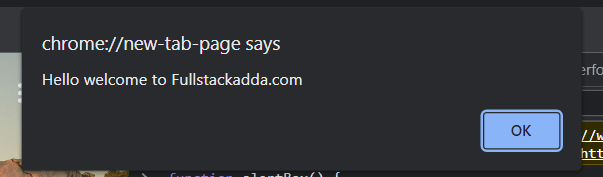
Function Return:
A return is a value that a function returns to the calling script or function when it completes its task. A return value can be any one of the four variable types: handle, integer, object, or string.
let x = calculate(4, 3);
function calculate(a, b) {
return a * b; // Function returns 12
}
There are three ways of writing a function in JavaScript:
- Function Declaration
- Function Expression
- Arrow Function
Function Declaration :
A function declaration is a named hoistable function definition (and declaration). In other words, it must always have a name and the function is always hoisted (moved) to the top of the scope.
function msg() {
alert( "Hello" );
}
Example:
function total(a, b) {
return a + b;
}
total(10, 20);
Function Expression:
A function expression is an optionally named non-hoistable function definition. If the function expression is not named, it is called an anonymous function. When a function expression is named, it is syntactically identical to the function declaration but still acts like a function expression.
let msg = function() {
alert( "Hello" );
};
Example:
const total = (function total(a, b) {
return a + b;
});
There are many useses of function expression in javascript though it’s almost similar to function definition. If you would have seen Callback, you must have encountered with frequent uses of function expression in stead of function definition.
Here you go for some points which make the concept of function expression worthwhile in javascript:—
Point 1 :- it’s quite convenient to pass the name of variable rather than whole body of function as an argument of other function( concept of callback) .Thus, it does enhance the readability of the code.
Point 2: A function expression can be used as a IIFE (Immediately Invoked Function Expression) which runs as soon as it is defined.
Point 3 : Function expressions in JavaScript are not hoisted, unlike function declaration.
Hoisting: Declearative statement gets more priority at the time of interpretation of the code.
Point 4: Function expression does make Anonymous function useable every now and then.
Arrow Function:
Arrows functions were introduced in ES6 JavaScript, and allows us to write shorter function syntax. It has several benefits :-
- Arrow functions take the this from their surroundings (called lexical binding).
Example :-
// ES5 way
var result = {
name: "Hello",
data: function data() {
setTimeout(function() {
console.log(this.name);
}.bind(this), 5000);
}
};
// ES6 way with arrow functions
var result = {
name: "Hello",
data: function data() {
setTimeout(() => {
console.log(this.name);
}, 5000);
}
};
The arrow comes after the list of parameters and is followed by the function’s body. It expresses something like “this input (the parameters) produces this result (the body).
When there is only one parameter name, you can omit the parentheses around the parameter list. If the body is a single expression, rather than a block in braces, that expression will be returned from the function. So, these two definitions of square
do the same thing:
let sample = [1,2,3,4];
let demo = sample.map((item) => item * 5);
let demo2 = sample.filter((item) => item < 3);
let demo3 = sample.reduce((result, item) => result + item);


- Functions are the block of code designed statements that perform a particular task.
- Function starts with the keyword function, followed by the name of the function.
Functions in JavaScript Functions in JavaScript Functions in JavaScript Functions in JavaScript Functions in JavaScript Functions in JavaScript