HTML forms are used to collect input from users on a web page. They are created using the <form>
tag, and consist of various form elements, such as text fields, checkboxes, radio buttons, and submit buttons, that are enclosed within the form tag.
These form elements are defined using various HTML form tags such as <input>
, <textarea>
, <select>
, <option>
, and <button>
. The input elements can be grouped together within a <fieldset>
element, and can be labeled using the <label>
tag.
Forms can be used to submit data to a server for processing using the HTTP method specified in the action
attribute of the <form>
tag, either GET or POST. The input data is sent along with the request as part of the body or url.
Forms are commonly used to create user registration forms, login forms, search forms, and surveys, and many other types of interactions on the web.
Some important Form elements are
These are the following HTML <form> elements:
- <label>: It defines label for <form> elements.
- <input>: It is used to get input data from the form in various types such as text, password, email, etc by changing its type.
- <button>: It defines a clickable button to control other elements or execute a functionality.
- <select>: It is used to create a drop-down list.
- <textarea>: It is used to get input long text content.
- <fieldset>: It is used to draw a box around other form elements and group the related data.
- <legend>: It defines caption for fieldset elements.
- <datalist>: It is used to specify pre-defined list options for input controls.
Here are some examples:
Type | Description |
---|---|
<input type=”text”> | Displays a single-line text input field |
<input type=”radio”> | Displays a radio button (for selecting one of many choices) |
<input type=”checkbox”> | Displays a checkbox (for selecting zero or more of many choices) |
<input type=”submit”> | Displays a submit button (for submitting the form) |
<input type=”button”> | Displays a clickable button |
Why need of HTML Form
HTML forms are helpful if you want to collect some data from random users.
For example: If a user want to purchase some items on internet, he/she must fill the form such as shipping address and credit/debit card details so that item can be sent to the given address.
Example
<!DOCTYPE html>
<html>
<head>
<title>Title of your page</title>
</head>
<body>
<h2>Welcome To fullstackadda.com</h2>
<form>
<p>
<label>Username : <input type="text" /></label>
</p>
<p>
<label>Password : <input type="password" /></label>
</p>
<p>
<button type="submit">Submit</button>
</p>
</form>
</body>
</html>
Output
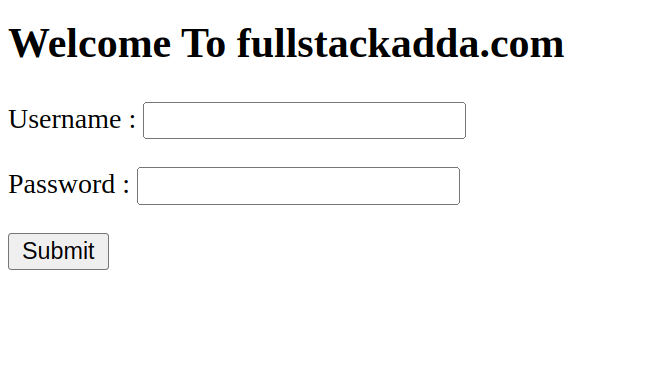
Radio Buttons in HTML
This is the syntax for to create a radio button in HTML <input type="radio">
Radio buttons are normally presented in radio groups (a collection of radio buttons describing a set of related options). Only one radio button in a group can be selected at the same time.
<!DOCTYPE html>
<html>
<head>
<title>Title of your page</title>
</head>
<body>
<h2>Welcome to fullstackadda.com</h2>
<h2>Select your gender</h2>
<form>
<label>Male<input type="radio" name="gender" value="male" /></label>
<label>Female<input type="radio" name="gender" value="female" /></label>
</form>
</body>
</html>
Output
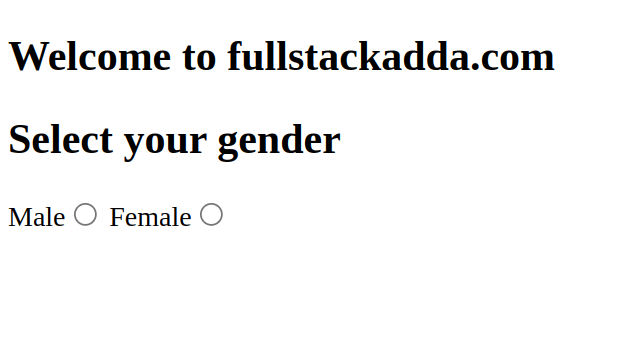
Checkbox in HTML
The <input type=”checkbox”> defines a checkbox. The checkbox is shown as a square box that is ticked (checked) when activated.
Checkboxes are used when there are lists of options and the user may select any number of choices, including zero, one, or several. In other words, each checkbox is independent of all other checkboxes in the list, so checking one box doesn’t uncheck the others.
Example
<!DOCTYPE html>
<html>
<head>
<title>Title of your page</title>
</head>
<body>
<h2>Choose your Country</h2>
<form>
<ul style="list-style-type:none;">
<li><input type="checkbox" name="country" value="India" />India</li>
<li><input type="checkbox" name="country" value="USA" />USA</li>
<li><input type="checkbox" name="country" value="Japan" />Japan</li>
</ul>
</form>
</body>
</html>
OUTPUT
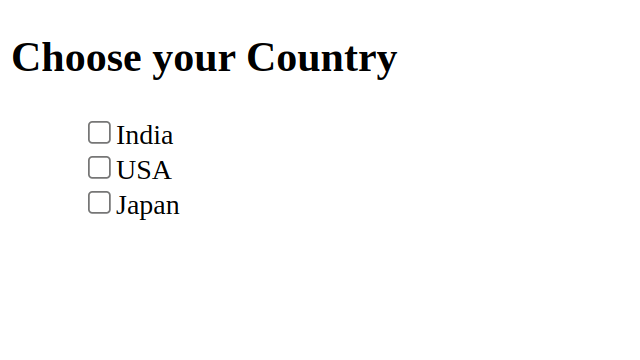

- HTML forms are used to collect input from users on a web page.
- HTML form tags such as
<input>
,<textarea>
,<select>
,<option>
, and<button>
.