Java Interview Questions and Answers
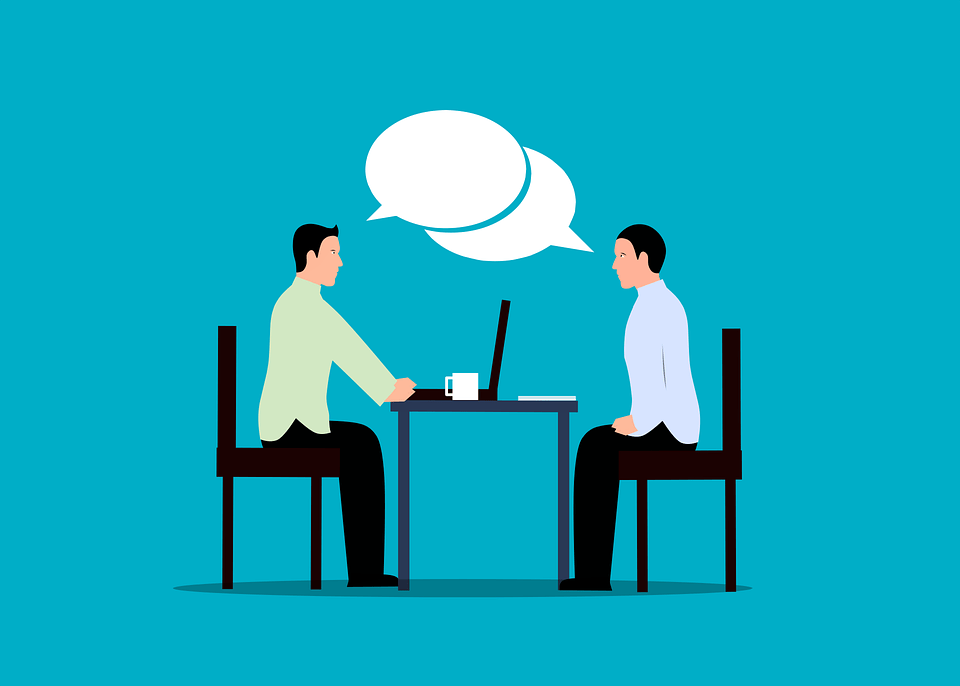
1. What is Java?
Java is a high-level programming language that was developed by James Gosling at Sun Microsystems in the mid-1990s. It is a platform-independent language that can be used to develop applications for desktop, mobile, and web platforms.
2.What are the features of Java?
Java has several features that make it a popular programming language, including platform independence, object-oriented programming, automatic memory management, multithreading, and robustness.
4. What is an object in Java?
An object in Java is an instance of a class that contains data and behavior. It is created from a class using the “new” keyword.
4. What is the difference between a class and an object?
A class is a blueprint or template for creating objects, while an object is an instance of a class.
5. What is inheritance in Java?
Inheritance is a mechanism in Java that allows a class to inherit properties and methods from another class. The class that is inherited from is called the superclass, and the class that inherits from it is called the subclass.
6. What is polymorphism in Java?
Polymorphism is a concept in Java that allows objects of different classes to be treated as if they were of the same class. This is achieved through method overriding and method overloading.
7. What is an interface in Java?
An interface in Java is a collection of abstract methods that can be implemented by any class. It defines a set of methods that a class must implement, but it does not provide the implementation of those methods.
8. What is a constructor in Java?
A constructor is a special method in Java that is used to initialize objects. It is called when an object of a class is created using the “new” keyword.
9. What is the difference between an abstract class and an interface?
An abstract class is a class that cannot be instantiated and may contain both abstract and non-abstract methods, while an interface is a collection of abstract methods that must be implemented by any class that implements it.
10. What is exception handling in Java?
Exception handling is a mechanism in Java that allows the programmer to handle runtime errors and exceptions that occur during the execution of a program. It involves catching the exception and taking appropriate actions to handle it, such as printing an error message or terminating the program.
11. What is the Java Virtual Machine (JVM)?
The JVM is an abstract computing machine that provides the runtime environment for Java programs. It interprets compiled Java code and executes it on the computer hardware.
12. What is a package in Java?
A package in Java is a mechanism for organizing classes and interfaces into namespaces. It helps to prevent naming conflicts and makes it easier to locate and use classes in a large project.
13. What is the difference between public, private, and protected access modifiers in Java?
Public, private, and protected are access modifiers in Java that determine the accessibility of class members. Public members can be accessed from anywhere, private members can only be accessed within the class, and protected members can be accessed within the class and its subclasses.
14. What is a static method in Java? Java Interview Questions and Answers
A static method in Java is a method that belongs to the class rather than to any specific instance of the class. It can be called using the class name, rather than an instance of the class.
15. What is a final keyword in Java?
The final keyword in Java can be used to indicate that a class cannot be extended, a method cannot be overridden, or a variable cannot be reassigned. It is often used to define constants.
16. What is method overloading in Java?
Method overloading is a feature in Java that allows a class to have multiple methods with the same name but different parameters. The correct method to call is determined by the number and types of the arguments passed to it.
17. What is method overriding in Java?
Method overriding is a feature in Java that allows a subclass to provide a specific implementation of a method that is already defined in its superclass. The signature of the method in the subclass must match that of the method in the superclass.
18. What is a thread in Java?
A thread in Java is a lightweight process that allows a program to execute multiple tasks concurrently. Each thread has its own call stack, but shares the same memory and resources as other threads in the program.
19. What is a synchronized keyword in Java?
The synchronized keyword in Java is used to control access to shared resources in a multithreaded environment. It ensures that only one thread can access the resource at a time, preventing race conditions and other synchronization problems.
20. What is a try-catch block in Java?
A try-catch block in Java is used for exception handling. The code that may throw an exception is placed in the try block, and any exceptions that are thrown are caught and handled in the catch block.
21. What is a stream in Java?
A stream in Java is a sequence of elements that can be processed in a functional programming style. It allows for efficient processing of large data sets and can be parallelized for improved performance.
22. What is the difference between checked and unchecked exceptions in Java?
Checked exceptions are checked at compile-time and must be handled by the programmer, while unchecked exceptions are not checked at compile-time and can be handled at runtime if necessary.
23. What is a lambda expression in Java?
A lambda expression in Java is a concise way to represent a functional interface, which is an interface with a single abstract method. It allows for functional programming in Java and simplifies code by removing boilerplate code.
24. What is a generic type in Java?
A generic type in Java is a class or interface that can work with any data type. It allows for code reusability and type safety.
25. What is the difference between an ArrayList and a LinkedList in Java?
An ArrayList is backed by an array and provides fast random access but slow insertion and deletion, while a LinkedList is backed by a doubly-linked list and provides fast insertion and deletion but slow random access.
26. What is a TreeMap in Java?
A TreeMap in Java is a collection that stores key-value pairs in a sorted order based on the keys. It allows for efficient searching and retrieval of elements.
27. What is a HashSet in Java?
A HashSet in Java is a collection that stores elements in an unordered manner, using a hash table to store and retrieve elements. It is useful for removing duplicates and checking for membership.
28. What is the difference between a HashMap and a Hashtable in Java?
A HashMap is not synchronized and allows null keys and values, while a Hashtable is synchronized and does not allow null keys or values.
29. What is the difference between a shallow copy and a deep copy in Java?
A shallow copy copies the references to objects, while a deep copy creates new objects and copies the values. A shallow copy can result in unexpected behavior if the original object is modified, while a deep copy is safer but may be slower.
30. What is the difference between an inner class and a nested class in Java?
An inner class is a non-static nested class that has access to the enclosing class’s members, while a nested class is any class that is defined inside another class.
31. What is a volatile keyword in Java?
The volatile keyword in Java is used to indicate that a variable’s value may be modified by multiple threads. It ensures that all threads see the most up-to-date value of the variable.
32. What is the difference between a stack and a queue in Java?
A stack is a data structure that follows the Last-In-First-Out (LIFO) principle, while a queue follows the First-In-First-Out (FIFO) principle.
33. What is a binary tree in Java?
A binary tree in Java is a data structure that consists of nodes connected by edges. Each node has at most two child nodes.
34. What is a binary search tree in Java?
A binary search tree in Java is a binary tree that has the property that the value of each node is greater than the values of all nodes in its left subtree and less than the values of all nodes in its right subtree.
35. What is a heap in Java?
A heap in Java is a data structure that stores elements in a way that allows for efficient retrieval of the minimum or maximum element.
36. What is a collection in Java?
A collection in Java is a group of objects that can be manipulated as a single unit. The Java Collections Framework provides a set of classes and interfaces for working with collections.
37. What is the difference between a set and a list in Java?
A set in Java is a collection that contains unique elements and does not preserve the order of elements, while a list is a collection that allows duplicates and preserves the order of elements.
38. What is the difference between a map and a set in Java?
A map in Java is a collection that maps keys to values, while a set is a collection that contains unique elements.
39. What is the difference between a private and a protected access modifier in Java?
A private access modifier in Java restricts access to the member to only within the same class, while a protected access modifier allows access to the member within the same class and its subclasses.
40. What is the difference between a static and a non-static method in Java?
A static method in Java is a method that belongs to the class and can be called without an instance of the class, while a non-static method is a method that belongs to an instance of the class.
41. What is an assertion in Java?
An assertion in Java is a statement that checks a condition at runtime and throws an error if the condition is false. It is used for debugging and testing.
42. What is a package in Java?
A package in Java is a namespace that organizes a set of related classes and interfaces.
43. What is the difference between an applet and an application in Java?
An applet in Java is a small program that is executed within a web browser, while an application is a standalone program that is executed on the user’s machine.
44. What is the difference between serialization and deserialization in Java?
Serialization in Java is the process of converting an object into a stream of bytes, while deserialization is the process of converting a stream of bytes back into an object.
45. What is a reflection in Java?
Reflection in Java is the ability of a program to examine and modify the behavior of objects at runtime.
46. What is the purpose of a package in Java?
A package in Java is a way of organizing related classes and interfaces into a single unit, making it easier to manage and maintain large code bases. Packages also help to avoid naming conflicts between classes and provide a level of access control to the classes within them.
47. How does Java handle multithreading?
Java handles multithreading through the use of threads, which are separate paths of execution that run concurrently within a program. The Java runtime environment manages the threads and provides synchronization mechanisms to ensure that they can access shared resources safely and avoid conflicts. The “synchronized” keyword can be used to create synchronized blocks of code that can be accessed by only one thread at a time.
48. What is the difference between a private and a protected method in Java?
A private method can only be accessed within the same class, while a protected method can be accessed within the same class and any subclasses. Java Interview Questions and Answers
49. How is memory allocation managed in Java?
Memory allocation in Java is managed by the JVM, which allocates memory on the heap for objects and on the stack for local variables and method calls. Java Interview Questions and Answers
50. What is the purpose of the “this” keyword in Java?
The “this” keyword in Java refers to the current object and is used to refer to instance variables and methods within the class.
Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers Java Interview Questions and Answers