Here is a list of possible interview questions of JavaScript, along with answers to help you prepare for your interview:
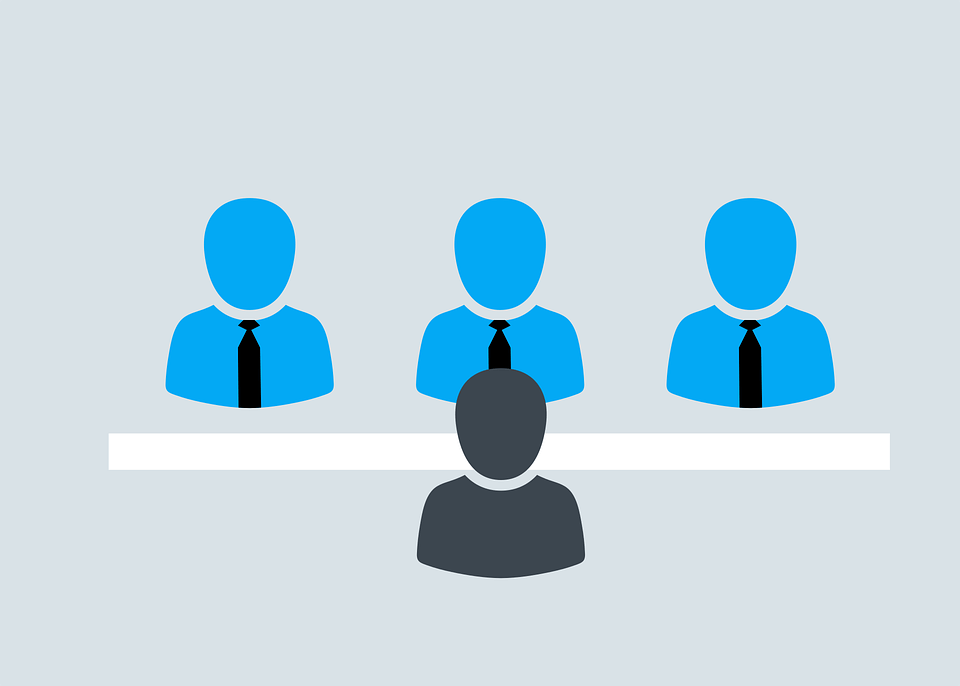
1. What is JavaScript, and what is it used for ?
JavaScript is a high-level, interpreted programming language that is commonly used to add dynamic and interactive elements to websites. It is used for a wide range of tasks, including form validation, creating animations, and manipulating the Document Object Model (DOM).
2. What is a variable in JavaScript, and how do you declare it ?
A variable in JavaScript is a container for storing data values. Variables can be declared using the var
, let
, or const
keywords, followed by the variable name.
For example:
var x;
let y;
const z = 10;
3. How to add JS to HTML?
- Three ways to add JavaScript to HTML pages.
- Embedding code
- Inline code
- External file
- Embedding code: To add the JavaScript code into the HTML pages, we can use the tag of the HTML that wrap around JavaScript code inside the HTML program.
- Inline code: Generally, this method is used when we have to call a function in the HTML event attributes.
- External file: We can also create a separate file to hold the code of JavaScript with the (.js) extension and later incorporate include it into our HTML document using the src attribute of the <script> tag.
4. What is an array in JavaScript, and how do you create one ?
An array in JavaScript is an ordered collection of data values, which can be of any data type, including numbers, strings, and objects. An array can be created using square brackets, []
, and elements can be separated by commas.
For example:
var numbers = [1, 2, 3, 4, 5];
var names = ['John', 'Jane', 'Jim'];
5. What is an object in JavaScript, and how do you create one ?
An object in JavaScript is an unordered collection of data values, which are stored as key-value pairs. An object can be created using curly braces, {}
, and properties can be added using key-value syntax.
For example:
var person = {
name: 'John Doe',
age: 30,
occupation: 'Developer'
};
6. What is Document in js?
Document in js:
- The document object represents our web page.
- If we want to access any element in an HTML page, we always start with accessing the document object.
7. Differences between JavaScript and java?
JavaScript | Java |
---|---|
JavaScript is a lightweight programming language(“scripting language”) and used to make web pages interactive. It can insert dynamic text into HTML. JavaScript is also known as the browser’s language. JavaScript(JS) is not similar or related to Java. Both the languages have a C like syntax and are widely used in client-side and server-side Web applications, but there are few similarities only. | Java is an object-oriented programming language and has a virtual machine platform that allows you to create compiled programs that run on nearly every platform. Java promised, “Write Once, Run Anywhere”. |
8. Is JavaScript Single-Threaded or Multi-Threaded?
JavaScript is a Single-Threaded Language, which means it has only one call stack that is used to execute the program.
9. Explain Garbage Collection in JavaScript?
- High-level languages, such as JavaScript, utilize a form of automatic memory management known as Garbage Collection (GC).
- The purpose of a garbage collector is to monitor memory allocation and determine when a block of allocated memory is no longer needed and reclaim it.
10. What is an event loop?
- The event loop is the secret behind JavaScript’s asynchronous programming.
- JS executes all operations on a single thread, but using a few smart data structures, gives us the illusion of multi-threading.
11. What is JavaScript Abstraction?
- JavaScript abstraction is a process of hiding the implementation details and displaying only the functionality to all the users.
- In simple words, we can say, JavaScript Abstraction ignores the irrelevant details and display only the necessary ones.
12. How to print in JS?
- You should use the console.log() method to print to console JavaScript. The JavaScript console log function is mainly used for code debugging as it makes the JavaScript print the output to the console
Example:
console.log("Hello World!");
13. Where do you write JavaScript?
- The HTML script tag is used to define JavaScript.
- The script element either contains script statements, or it points to an external script file through the src attribute.
14. What is execution context?
Execution Context
- The environment in which JavaScript Code runs is called Execution Context.
- Execution context contains all the variables, objects, and functions.
- Execution Context is destroyed and recreated whenever we reload an Application.
15. How to print the output in JavaScript (not in the console)?
JavaScript can display data in different ways:
- Writing into an HTML element, using innerHTML.
- Writing into the HTML output using document.write().
- Writing into an alert box, using window.alert().
16. If JavaScript is synchronous why do we have asynchronous functions?
- Asynchronous JavaScript
- Many Web API features now use asynchronous code to run, especially those that access or fetch some kind of resource from an external device, such as fetching a file from the network, accessing a database, and returning data from it, accessing a video stream from a webcam, etc.
17. Explain about sprite in JavaScript?
- Sprite.js is a framework that lets you create animations and games efficiently using sprites.
- The goal is to allow a common framework for desktop and mobile browsers and use the latest technology available on each platform.
- Sprite.js is tested on WebKit, Firefox, Android phones, Opera, and IE9.
18. How do we read and write files in JavaScript?
Files can be read and written by using java script functions –
fopen() fread() and fwrite()
- fopen() : The function fopen() takes two parameters – 1. Path and 2. Mode (0 for reading and 3 for writing). The fopen() function returns -1 if the file is successfully opened.
Example
`file=fopen(getScriptPath(),0)`;
- fread() : The function fread() is used for reading the file content.
Example
`str = fread(file,flength(file)` ;
- fwrite() : The function fwrite() is used to write the contents to the file.
Example
`file = fopen("c:\MyFile.txt", 3);// opens the file for writing``fwrite(file, str);// str is the content that is to be written into the file.`;
19. Write an example program for class?
Example
class User {
constructor(name) {
this.name = name;
}
sayHi() {
alert(this.name);
}
}
// Usage:
let user = new User("John");
user.sayHi();
20. What language is js?
- JavaScript is known as the scripting language for Web pages.
- It runs on the client-side of the web, which can be used to design/program how the web pages behave on the occurrence of an event. JavaScript is an easy to learn and also a powerful scripting language, widely used to add interactivity to the webpage.
21. Why JavaScript is called as a loosely typed or a dynamic language?
- JavaScript is called a loosely typed or a dynamic language because JavaScript variables are not directly associated with any value type and any variable can be assigned and re-assigned values of all types:
Example:
let myvar = "abc"; // myvar is string
myvar = true; // myvar is now a boolean
myvar = 10; // myvar is now a number
22. List some of the advantages of JavaScript.
- Server interaction is less.
- Feedback to the visitors is immediate.
- Interactivity is high.
23. What is the output of 10+20+”30″ in JavaScript?
3030
24. List down some of the features of ES6
Below is the list of some new features of ES6
- Support for constants or immutable variables
- Block-scope support for variables, constants, and functions
- Arrow functions
- Default parameters
- Rest and Spread Parameters
- Template Literals
- Multi-line Strings
- Destructuring Assignment
- Enhanced Object Literals
- Promises
- Classes
25. What is ES6?
ES6 is the sixth edition of the JavaScript language and it was released in June 2015. It was initially known as ECMAScript 6 (ES6) and later renamed ECMAScript 2015. Almost all the modern browsers support ES6 but for the old browsers, there are many transpilers, like Babel.js, etc.
26. Data structures in JavaScript?
- JavaScript has primitive and non-primitive data structures. Primitive data structures and data types are native to the programming language. These include boolean, null, number, string, etc.
- Non-primitive data structures are not defined by the programming language but rather by the programmer. These include linear data structures, static data structures, and dynamic data structures, like queue and linked lists.
27. Is JS single threaded or multi threaded?
- JavaScript is a single-threaded language.
- A single-thread language is one with a single call stack and a single memory heap. It means that it runs only one thing at a time.
28. How to convert a string to a number?
- The parseInt() function accepts a string and converts it into an integer.
29. Explain Implicit Type Coercion in JavaScript.
- Implicit type coercion in JavaScript is automatic conversion of value from one data type to another. It takes place when the operands of an expression are of different data types.
Example:
var x = 3;
var y = "3";
console.log(x + y); // Returns "33"
30. What is an alert msg in JS?
alert() instructs the browser to display a dialog with an optional message, and to wait until the user dismisses the dialog.
31. Is the alert box and confirmation box are similar?
No, they are not the same. Their difference can be found in the button. An alert box contains only one OK button whereas a confirmation box contains two buttons i.e. Cancel and OK.
32. How do you debug errors in JavaScript?
- Using console.log() Method
- Setting Breakpoints or using Debugger in code
- Using Browser Debugging tools
- To know more, refer to below references
33. Explain about closures in JavaScript?
- Closures is a combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment).
- In other words, a closure gives you access to an outer function’s scope from an inner function. In JavaScript, closures are created every time a function is created, at function creation time.
Example:
function makeFunc() {
let name = "ShivaSingam";
function displayName() {
alert(name);
}
return displayName;
}
let myFunc = makeFunc();
myFunc();
34. How do we submit a form in js?
The submit() method submits the form in js by clicking the HTML button element with type submit.
35. How many scopes of a variable does JavaScript have?
- JavaScript has two scopes of any variable, local and global.
- Local Scope: A variable having local scope can be assessed only within the function where it is defined. Parameters of the functions are local for the variable.
- Global Scope: A variable having global scope is visible and accessible anywhere in the JavaScript code.
36. What are functions hoisting and variables hoisting?
- Hoisting is a JavaScript technique that moves variables and function declarations to the top of their scope before code execution begins.
Example:
console.log(functionBelow("Hello"));
function functionBelow(greet) {
return `${greet} world`;
}
console.log(functionBelow("Hi"));
37. What are the differences between class and object?
Class | object |
---|---|
Class is a template for creating objects in a program | Object is an instance of a class |
Class is a logical entity | Object is a physical entity |
Class does not allocate memory space | Object allocates memory space |
You can declare class only once | You can create more than one object using a class |
Classes can’t be manipulated | objects can be manipulated |
Classes doesn’t have any Values | Objects have its own values |
You can create a class using the “class” keyword | You can create an object using the “new” keyword in JavaScript |
38. Define class and object?
Class:
- The Class is a special type of function used for creating multiple objects.
Syntax:
class ClassName {
constructor() {}
method_1() {}
method_2() {}
method_3() {}
}
Object:
- An Object is a collection of properties.
- A property is an association between a name (or key) and a value.
- For example, a person has a name, age, city, etc. These are the properties of the person.
let person = {
firstName: "Shiva",
lastName: "Singam",
age: 24,
};
39. Explain about object, class, and init method in JS?
- Object: A JavaScript object is an entity having state and behavior (properties and method). For example car, pen, bike, chair, glass, keyboard, monitor, etc. JavaScript is an object-based language. Everything is an object in JavaScript.
- Class: Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
- init() method: JavaScript doesn’t have a built-in init() function, that is, it’s not a part of the language. But it’s not uncommon (in a lot of languages) for individual programmers to create their own init() function for initialization stuff.
Submit Feedback
40. Explain global and local variables in JavaScript?
Global | Local |
---|---|
local variables are variables that are defined within functions. They have local scope, which means that they can only be used within the functions that define them. | Global variables are variables that are defined outside of functions. These variables have global scope, so they can be used by any function without passing them to the function as parameters. |
41. Explain the difference between var and let?
- The main difference between let and var is that scope of a variable defined with let is limited to the block in which it is declared while the variable declared with var has the global scope. So we can say that var is rather a keyword that defines a variable globally regardless of block scope.
- The scope of let not only limited to the block in which it is defined but variable with let also do not get added with global window object even if it get declared outside of any block. But we can access variables with var from the window object if it is defined globally.
42. What is a static variable in JS?
- A static variable is a class property that is used in a class and not on the instance of the class. To use a static variable, we use the static keyword. The value of a static variable is set at the run time and is a kind of global value that can be used for the instance of the specified class.
- If you declare any variable as static, it is known as a static variable. The static variable can be used to refer to the common property of all objects (which is not unique for each object), for example, the company name of employees, college name of students, etc.Syntax: static string college =”ITS”; //static variable
43. What is the difference between let, const, var?
NAME | DESCRIPTION |
---|---|
let | The let statement declares a block-scoped local variable, optionally initializing it to a value. |
const | Constants are block-scoped, much like variables declared using the let keyword. The value of a constant can’t be changed through reassignment, and it can’t be redeclared. |
var | The var statement declares a function-scoped or globally-scoped variable, optionally initializing it to a value. |
44. What is the difference between let and const variables in JavaScript?
let | const |
---|---|
let is now preferred for variable declaration. It’s no surprise as it comes as an improvement to var declarations. | Variables declared with the const maintain constant values. const declarations share some similarities with let declarations. |
let is block scoped. | const declarations are block-scoped. |
let can be updated but not re-declared. | const cannot be updated or re-declared. |
45. What are variables?
Variables are like containers for storing values. Values in the variables can be changed.
46. What are global variables?
Global variables are those that are available throughout the length of the code without any scope.
47. Explain data types in JavaScript?
- JavaScript has different data types
- Primitive
- Non-Primitive.
- There are seven primitive data types
- number
- string
- boolean
- null
- undefined
- symbol
- There is one non-primitive data type
- object
48. Explain about undefined in JavaScript?
Undefined
- If a value is not assigned to the variable, then it takes undefined as its value.
- In JS, undefined refers to the value that is not being assigned.
49. What are primitive data types in JavaScript?
Primitive Types
- number
- string
- boolean
- null
- undefined
- symbol
51. Explain about Symbols in JavaScript?
- Symbols are a new primitive type introduced in ES6. Symbols are unique identifiers. Just like their primitive counterparts (number, string, boolean), they can be created using the factory function Symbol() which returns a Symbol.
- Symbols are often used to add unique property keys to an object that won’t collide with keys any other code might add to the object, and which are hidden from any mechanisms other code will typically use to access the object. That enables a form of weak encapsulation or a weak form of information hiding.
52. What is NaN property in JavaScript?
- NaN property represents Not-a-Number value. It indicates a value that is not a legal number.
- typeof of a NaN will return a Number.
- To check if a value is NaN, we use the isNaN() function.
Example:
isNaN(345); // Returns false
isNaN(undefined); // Returns true
53. What is the difference between an undefined value and a null value?
- Undefined value: A value that is not defined and has no keyword is known as an undefined value.Example:1letnumber;//Here, a number has an undefined value.JAVASCRIPT
- Null value: A value that is explicitly specified by the keyword null is known as a null value.
Example:
let number; //Here, a number has an undefined value.
Null value: A value that is explicitly specified by the keyword null is known as a null value.
Example:
let str = null; //Here, str has a null value.
54. What is the use of the Split method?
The split() method is used to split a string into an array of substrings and returns the new array.
Example:
const string = "How are you doing today?";
const result = string.split(" ");
console.log(result);
Output:
["How", "are", "you", "doing", "today?"]
55. What is the use of the Join method?
- The join() method returns the array as a string.
- The elements will be separated by a specified separator. The default separator is the comma (,).
const fruits = ["Banana", "Orange", "Apple", "Mango"];
const energy = fruits.join();
Output:
Banana,Orange,Apple,Mango
Note
This method will not change the original array.
56. What are the ways to define a variable in JavaScript?
Here are 3 ways to define a variable:
- var: The JavaScript variables statement is employed to declare a variable and, optionally, we will initialize the worth of that variable. For instance: var x =60; Before executing the code, all the variable declarations are processed at first.
- const: The thought of const functions doesn’t allow them to switch the thing on which they’re called. When a function is said as const, it is often called on any sort of object.
- let: It is a signal that the variable may be reassigned, such as a counter in a loop, or a value swap in an algorithm. It also signals that the variable is going to be used only within the block it’s defined in.
57. Example for addition and subtraction in JavaScript?
- The addition operator (+) adds numbers
Example:
const x = 5;
const y = 2;
const z = x + y;
- The subtraction operator (-) subtracts numbers
Example:
const x = 5;
const y = 2;
const z = x - y;
58. What is the difference between loose equal to (==) and strict equal to (===)?
- Loose equal to (==)
- Loose equality compares two values for equality but doesn’t compare types of values.
- Strict equal to (===)
- Strict equality compares two values for equality including types of values.
Example:
console.log(2 == "2"); // true
console.log(2 === "2"); // false
59. What is a Switch case?
- The switch statement executes a block of code depending on different cases.
- It evaluates an expression. The value of the expression is then compared with the values of each case in the structure. If there is a match, the associated block of code is executed.
Example:
switch(expression) {
case n:
code block
break;
case n:
code block
break;
default:
default code block
}
- The break keyword stops the execution.
- The default keyword specifies some code to run if there is no case match. There can only be one default keyword in a switch.
60. What is the difference between the Spread operator and Rest parameter?
- Spread Operator: The Spread Operator is used to unpack an iterable (e.g. an array, object, etc.) into individual elements.
Example:
let arr1 = [2, 3];
let arr2 = [1, ...arr1, 4];
console.log(arr2); // [1, 2, 3, 4]
- Rest Parameter: With Rest Parameter, we can pack multiple values into an array.
Example:
function numbers(...args) {
console.log(args); // [1, 2, 3]
}
numbers(1, 2, 3);
61. Explain about Loops in JavaScript.
- In JavaScript, Loops are used to execute a block of code repeatedly. Loops offer a quick and easy way to repeat an action. They execute the same lines of code a specific number of times or as long as a specific condition is true.
- JavaScript supports the following types of loops:
- for loop: loops through a block of code several times
Syntax:
for(statement1; statement2; statement3)
{
//lines of code to be executed
}
- for-in loop: It is used to loop the object through properties.
Syntax:
for (variablename in objectname)
{
//lines of code to be executed
}
- while loop: loops through a block of code while a specified condition is true.
Syntax:
while(condition)
{
//lines of code to be executed
}
- do/while loop: loops through a block of code while a specified condition is true. It is similar to the while loop. The only difference is that in the do/while loop, the block of code gets executed once even before checking the condition.
Syntax:
do
{
//block of code to be executed
} while (condition
62. What is the output for add 10+”10″ in js?
const result = 10 + "10";
console.log(result); // output: 1010
console.log(typeof result); // output : string
63. What is implicit conversion in JavaScript?
- In certain situations, JavaScript automatically converts one data type to another (to the right type). This is known as implicit conversion.
Example:
let result;
result = "3" + 2;
console.log(result); // output: 32
result = "3" + true;
console.log(result); // output: 3true
- NoteWhen a number is added to a string, JavaScript converts the number to a string before concatenation.
64. What is the output for add 10+10 in js?
const result = 10 + 10;
console.log(result); // output: 20
console.log(typeof result); // output : number
65. Explain break and continue statements?
Break statement | Continue statement |
---|---|
The break statement is used to jump out of a loop. It can be used to jump out of a switch() statement. It breaks the loop and continues executing the code after the loop. | The continue statement jumps over one iteration in the loop. It breaks iteration in the loop and continues executing the next iteration in the loop. |
66. Why does it return false when comparing two similar objects in JavaScript?
- Suppose we have an example below.
let a = { a: 1 };
let b = { a: 1 };
let c = a;
console.log(a === b); // output: false (even though they have the same property)
console.log(a === c); // output: true
67. What does the || operator do?
- The || or Logical OR operator finds the first truthy expression in its operands and returns it. This too employs short-circuiting to prevent unnecessary work.
Example:
console.log(null || 1 || undefined); // output: 1
68. What happens in the following scenario “3”+3, “3”-3?
- When the + operator is used, if any operand is a string, string concatenation is performed.
- When the – operator is used then all operands are converted to numbers.
console.log("3"+3); //output: 33
console.log("3"-3); //output: 0
69. What is the difference between if else and switch statements?
if-else | switch |
---|---|
Depending on the condition in the ‘if’ statement, ‘if’ and ‘else’ blocks are executed. | The user will decide which statement is to be executed. |
It contains either logical or equality expression. | It contains a single expression which can be either a character or integer variable. |
First, the condition is checked. If the condition is true then ‘if’ block is executed otherwise ‘else’ block | It executes one case after another till the break keyword is not found, or the default statement is executed. |
If the condition is not true, then by default, else block will be executed. | If the value does not match with any case, then by default, default statement is executed. |
If there are multiple choices implemented through ‘if-else’, then the speed of the execution will be slow. | If we have multiple choices then the switch statement is the best option as the speed of the execution will be much higher than ‘if-else’. |
70. Explain about Arrays in JavaScript?
JavaScript Arrays are used to store multiple values in a single variable. Arrays are a special type of object. The
typeof operator in JavaScript returns
object for arrays.
71. How to compare two arrays?
- In JavaScript, to compare two arrays we need to check that the length of both arrays should be the same.
- The objects present in it are of the same type and each item in one array is equal to the counterpart in another array.
- By doing this we can conclude both arrays are the same or not.
- JavaScript provides a function JSON.stringify() in order to convert an object or array into JSON string. By converting into JSON string we can directly check if the strings are equal or not.
72. In JavaScript, if you want to create a similar architecture like a tuple what will you do?
- In JavaScript, arrays allow multiple types to be grouped. For our purposes, a tuple in JavaScript will simply be an array of known length, whose values are of a known type, such as an array of three numbers ( example : [45, 30, 90] ).
73. Initial quantity of array list?
- The initial or default quantity of an Array List is 10. It means when we create an ArrayList without specifying any quantity, it will be created with the default capacity, i.e., 10. Hence, an Array List with the default capacity can hold ten (10) values.
74. How can you create an Array in JavaScript?
- We can define arrays using the array literal as follows
let x = [];
let y = [1, 2, 3, 4, 5];
75. Explain about reduce, map, filter methods?
- reduce() method executes a reducer function (that you provide) on each element of the array, resulting in a single output value.
- Syntax: array.reduce(function(accumulator, currentValue, index, arr), initialValue)
- Map() method creates a new array with the results of calling a function for every array element.
- The map() method calls the provided function once for each element in an array, in order.
- Syntax: array.map(callback(currentValue, index, arr))
- filter() method creates a new array filled with all elements that pass the test (provided as a function).
- A new array with the elements that pass the test will be returned. If no elements pass the test, an empty array will be returned.
- Syntax: array.filter(function(currentValue, index, arr))
76. What is the use of the push() method in JavaScript?
- The push() method adds new items to the end of the array.
77. Write a sample program for map and filter?
- Sample program for map:
const numbers = [1, 2, 3, 4];
const doubled = numbers.map((item) => item * 2);
console.log(doubled); // [2, 4, 6, 8]
- Sample program for filter:
const numbers = [1, 2, 3, 4];
const evenNumbers = numbers.filter((item) => item % 2 === 0);
console.log(evenNumbers); // [2, 4]
78. In JavaScript write use of map function?
Use of map function:
- Instead of manually iterating over the array using a loop, we can simply use the built-in Array.map() method.
- The Array.map() method allows you to iterate over an array and modify its elements using a callback function. The callback function will then be executed on each of the array’s elements.
79. Explain about list slice?
- The slice() method returns the selected elements in an array, as a new array object.
- The slice() method selects the elements starting at the given start index, and ends at, but does not include the given end index.
Example:
let fruits = ["Banana", "Orange", "Lemon", "Apple", "Mango"];
let citrus = fruits.slice(1, 3); //Orange,Lemon
80. How do you empty an array??
- There are several ways to do this, probably the simplest is to reassign the variable to an empty array.
Example:
let array = [1, 2, 3];
// Assigning to an empty array
array = [];
// Setting its length property to 0
array.length = 0;
// Using splice with the array's length
array.splice(0, array.length);
81. What is an object and explain object creation?
- An object is a collection of properties, and a property is an association between a name (or key) and a value.
- In JavaScript, there are four methods to use to create an object:
- Object Literals An object literal also called an object initializer, is a comma-separated set of paired names and values. We can create an object literal as shown below
const car = {
model: "BMW",
color: "red",
price: 2000,
};
- New operator or constructor Using the constructor function. If we call a function using a new operator, the function acts as a constructor and returns an object. Consider the following code:
function Car(model, color) {
this.model = model;
this.color = color;
}
let c1 = new Car("BMW", "red");
- Object.create method We can also create new objects using the Object.create() method, which allows us to specify the prototype object and the properties. For example:
let Car = {
model: "BMW",
color: "red",
};
- We can use the Car object as a prototype to create another object, as shown below:
let ElectricCar = Object.create(Car);
- ClassUsing class keyword. Now we can use the class attribute to create a class in JavaScript instead of a function constructor, and use the new operator to create an instance. Consider the following code:
class Car {
constructor(maker, price) {
this.maker = maker;
this.price = price;
}
getInfo() {
console.log(this.maker + " costs : " + this.price);
}
}
- We can use the Car class to create objects as shown below:
let car1 = new Car("BMW", 100);
car1.getInfo();
let car2 = new Car("Audi", 150);
car2.getInfo();
82. What are object prototypes?
All JavaScript objects inherit properties from a prototype.
- Date objects inherit properties from the Date prototype.
- Math objects inherit properties from the Math prototype.
- Array objects inherit properties from the Array prototype.
- A prototype is a blueprint of an object. The prototype allows us to use properties and methods on an object even if the properties and methods do not exist on the current object.
Example:
var arr = [];
arr.push(2);
console.log(arr);
83. How to add key value pair to array in JavaScript?
There are two possible solutions to add new properties to an object.
- Using dot notation: This solution is useful when you know the name of the property.
Example:
var arr = [];
arr.push(2);
console.log(arr);
- Using square bracket notation: This solution is useful when the name of the property is dynamically determined.
Example:
let object = {
key1: value1,
key2: value2,
};
obj["key3"] = "value3";
84. What is Object Destructuring?
- To unpack properties from Objects, we use Object Destructuring. The variable name should match with the key of an object.
Example:
let person = {
firstName: "Shiva",
lastName: "Singam",
age: 28,
};
let { age } = person;
console.log(age); // 28
85. Why do we use this keyword in the Arrow function?
- In arrow functions, there is no need to bind this.
- The this keyword always represents the object that is defined in the arrow function.
86. What does the this operator in JavaScript do?
The this keyword used in JavaScript talks about the object which it belongs. It has many different values and it depends on where it is being used.
87. How many JavaScript array methods are there and which are the most useful?
There are 30 JavaScript array methods. Among the most useful are:
- toString()
- is used with a number and converts the number to a string.
- pop() and push()
- pop() method removes the last element of an array and returns it and push() method adds an element to an array at last position.
- shift() and unshift()
- shift() method removes the first element of an array and unshift() method adds one element to the beginning of an array.
- splice()
- is used to add or remove array items
- concat()
- is used to merge arrays
- slice()
- slice out part of an array and put it in a new array
- forEach()
- loop through array
- filter()
- create a new array with chosen elements
- map()
- make a new array based on a function
- sort()
- rearrange the array order
88. What are the differences between JavaScript array methods slice and splice?
Slice | Splice |
---|---|
Doesn’t modify the original array(immutable) | Modifies the original array(mutable) |
Returns the subset of original array | Returns the deleted elements as array |
Used to pick the elements from array | Used to insert or delete elements to/from array |
89. Explain the pop() and shift() methods in JavaScript?
- The pop() method removes the last element from an array and returns it. The array on which it is called is then altered.
- The shift() method removes an item from the beginning of an array and returns the removed item.
- In both methods, the length of the array is changed. The return value of the pop and shift methods is the removed item.
- Pop Example: Remove the last element of an array:
let fruits = ["Apple", "Orange", "Banana", "Strawberry"];
fruits.pop(); // output: ["Apple", "Orange", "Banana"]
- Shift Example: Remove the first element of an array:
let fruits = ["Apple", "Orange", "Banana", "Strawberry"];
fruits.shift(); // output: ["Orange", "Banana", "Strawberry"]
90. What is the difference between map() and forEach()?
Both of them iterate through the elements of an array. The difference here is that the map creates a new array while forEach doesn’t.
91. How to get all keys of an object in JavaScript?
The easy way is using
Object.keys(). This will return an array of given object’s own enumerable properties.
92. Which JavaScript methods are used to search an element in an array?
Array Methods
- The methods arr.indexOf, arr.lastIndexOf and arr.includes have the same syntax and do essentially the same as their string counterparts, but operate on items instead of characters:
- arr.indexOf(item, from) looks for item starting from index from, and returns the index where it was found, otherwise -1.
- arr.lastIndexOf(item, from) – same, but looks for from right to left.
- arr.includes(item, from) – looks for item starting from index from, returns true if found.
- find: The find method looks for a single (first) element that makes the function return true.
- findIndex: The arr.findIndex method is essentially the same, but it returns the index where the element was found instead of the element itself and -1 is returned when nothing is found.
- filter: The filter method looks for elements that make the function return true.
93. What is the purpose of the array splice method?
- The splice() method is used either adds/removes items to/from an array, and then returns the removed item.
- The first argument specifies the array position for insertion or deletion whereas the optional second argument indicates the number of elements to be deleted. Each additional argument is added to the array.
Example:
let arrayIntegersOriginal1 = [1, 2, 3, 4, 5];
let arrayIntegersOriginal2 = [1, 2, 3, 4, 5];
let arrayIntegersOriginal3 = [1, 2, 3, 4, 5];
let arrayIntegers1 = arrayIntegersOriginal1.splice(0, 2); // output: [1, 2]; original array: [3, 4, 5]
let arrayIntegers2 = arrayIntegersOriginal2.splice(3); // output: [4, 5]; original array: [1, 2, 3]
let arrayIntegers3 = arrayIntegersOriginal3.splice(3, 1, "a", "b", "c"); // output: [4]; original array: [1, 2, 3, "a", "b", "c", 5]
94. How to sort the numbers in an array?
By default, the sort method sorts elements alphabetically. To sort numerically just add a compare method that handles numeric sorts:
Example:
let numArray = [140000, 104, 99]; numArray.sort(function(a, b) { return a - b; }); console.log(numArray);
95. How do you flatten a multidimensional array?
The
flat() method creates a new array with all sub-array elements concatenated into it recursively up to the specified depth.
Example:
const arrToConvert=[4,2,[23,14,[65,16,[87,[18,99,110]]]]];
function get1DArray(arr){
return arr.flat(4);
}
console.log(get1DArray(arrToConvert)); //Output: [4, 2, 23, 14, 65, 16,87, 18, 99, 110]
96. How to write a 2D array?
- The syntax of a multidimensional array is the same as the one-dimensional array:
Example:
let myDayPlan = [ ['Work', 9], ['Eat', 1], ['Commute', 2], ['Play Game', 1], ['Sleep', 7] ];
97. Explain JavaScript functions and their uses?
- A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when it is invoked (called).
- Define the code once, and use it many times. You can use the same code many times with different arguments, to produce different results (You can reuse code).Example:123functionmyFunction(p1, p2){returnp1*p2;// The function returns the product of p1 and p2}JAVASCRIPT
98. What is a self-invoking function?
- The self-invoking function in JavaScript is known as Immediately Invoked Function Expressions(IIFE).
- Immediately Invoked Function Expressions (IIFE) is a JavaScript function that executes immediately after it has been defined so there is no need to manually invoke IIFE.
99. What are the differences between general functions and arrow functions?
In JavaScript, there are two main ways to create functions: using the “function” keyword and using arrow functions.
General functions are created using the “function” keyword. They are used to create named or anonymous functions that can be reused throughout the code. They have their own “this” binding, meaning that the value of “this” inside the function can be different from the value of “this” outside of the function. They also have their own “arguments” object, which allows you to access the arguments passed to the function.
Arrow functions, on the other hand, are created using the “=>” operator. They are a shorthand way to create anonymous functions and are often used in callback functions, event handlers, and when defining object methods. Unlike general functions, arrow functions do not have their own “this” binding. Instead, they inherit the “this” binding of the surrounding code. This means that the value of “this” inside an arrow function will be the same as the value of “this” outside of the function. Arrow functions also do not have their own “arguments” object and instead you can use the rest parameter to access the arguments passed to the function.
Examples:
General function:
function add(a, b) {
return a + b;
}
Arrow Function
const add = (a, b) => {
return a + b;
}
OR
const add = (a, b) => a + b;
In summary, the main difference between general functions and arrow functions is the way they bind the “this” keyword and the way they access the arguments passed to the function. Arrow functions are generally considered more concise and are often used in functional programming.
100. Explain JavaScript functions?
- A JavaScript function is a block of code designed to perform a particular task. A JavaScript function is executed when something invokes it (calls it).
- Define the code once, and use it many times. You can use the same code many times with different arguments, to produce different results (You can reuse code ).