Here is a list of possible interview questions of Python, along with answers to help you prepare for your interview:
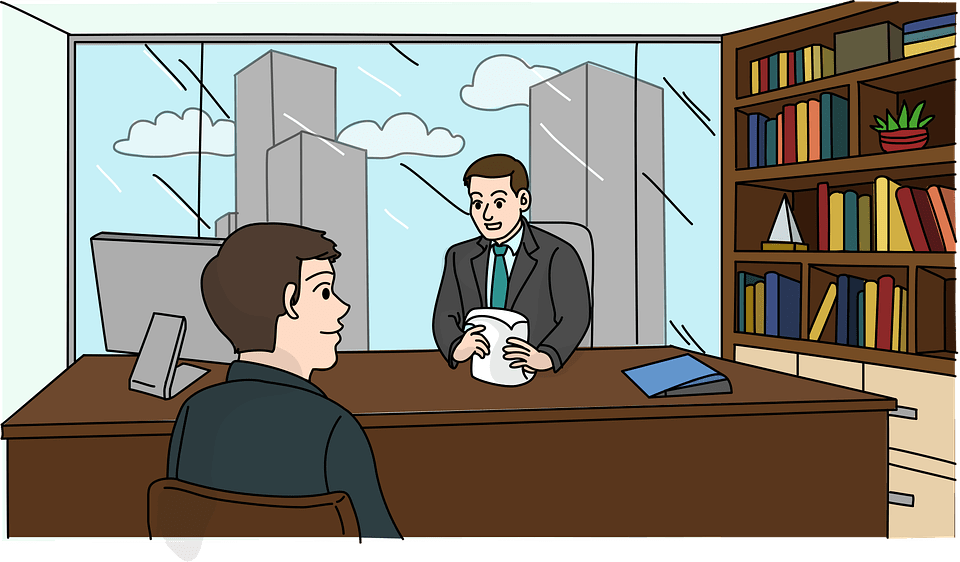
Beginner Level ———-
1. What is Python used for?
Python is a general-purpose programming language that is widely used for web development, scientific computing, data analysis, artificial intelligence, and many other areas.
2. What are the key features of Python?
- Easy to learn and read
- Interpreted, not compiled
- Dynamically-typed
- High-level, object-oriented language
3. What are some data types in Python?
- int (integer)
- float (floating point number)
- str (string)
- list
- tuple
- dict (dictionary)
CLICK HERE for more information
4. How do you create a variable in Python?
- Variables are created by assigning a value to a name using the assignment operator (=).
5. What is the difference between a list and a tuple?
- A list is mutable (can be changed), while a tuple is immutable (cannot be changed).
6. How do you create a function in Python?
- Functions are created using the “def” keyword, followed by the function name and parentheses.
7. What is the difference between “==” and “is”?
- “==” compares the values of two objects, while “is” compares the memory locations of two objects.
8. What is the difference between a local variable and a global variable?
- Local variables are only accessible within the function or block of code where they are defined, while global variables can be accessed from anywhere in the program.
9. How do you handle exceptions in Python?
- Exceptions are handled using try-except blocks.
10. What is the difference between a module and a package?
- A module is a single Python file, while a package is a collection of modules.
11. How do you create a class in Python?
In Python, classes are created using the class
keyword, followed by the class name and a colon. The class definition should be indented, and all the methods and properties of the class should be defined within this indented block.
Here’s an example of how to create a simple class called “Person”:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print("Hello, my name is {} and I am {} years old.".format(self.name, self.age))
12. What is inheritance in Python?
Inheritance is a mechanism in object-oriented programming (OOP) that allows a new class to be defined by inheriting properties and methods from an existing class. The existing class is called the base class or the parent class, and the new class is called the derived class or the child class.
Inheritance enables code reuse and promotes the DRY (Don’t Repeat Yourself) principle in programming. By inheriting from a base class, a derived class can inherit the attributes and behaviors of the base class, and can also add new attributes and behaviors of its own.
In python, you can use the class DerivedClassName(BaseClassName)
syntax to create a derived class that inherits from a base class. The base class is specified in the parentheses after the derived class name.
CLICK HERE for more information
13. What is Polymorphism in Python?
14. What is Encapsulation in Python?
15. What is the difference between a for loop and a while loop in Python?
Here is a table that compares the for
loop and the while
loop in Python:
for loop | while loop |
---|---|
The for loop is used to iterate over a sequence (such as a list, tuple, or string) or other iterable object. | The while loop is used to repeatedly execute a block of code as long as a certain condition is true. |
The for loop has a definite iteration, that is, the loop variable takes on all the values in the sequence. | The while loop has an indefinite iteration, that is, the loop variable takes on values until the condition is no longer true. |
The for loop is best used when you know the number of iterations in advance. | The while loop is best used when you don’t know the number of iterations in advance. |
The for loop is less prone to errors since it is definite iteration. | The while loop is more prone to errors since it is indefinite iteration and if the condition is never met then the loop will run indefinitely. |
16. Difference between list and tuple ?
Lists | Tuples |
---|---|
Mutable (can be modified) | Immutable (cannot be modified) |
Created with square brackets [] | Created with round parentheses () or the tuple() function |
Can contain multiple data types | Can contain multiple data types |
Can be used as an argument in functions that modify the list (e.g. list.append()) | Can be used as a key in a dictionary |
17. What is the use of the range() function in Python ?
The range()
function in Python is used to generate a sequence of numbers. The function takes three arguments: start
, stop
, and step
. The start
argument is the first number in the sequence, the stop
argument is the first number that is not in the sequence, and the step
argument is the difference between each number in the sequence.
For example, range(0, 10)
generates a sequence of numbers from 0 to 9, range(0, 10, 2)
generates a sequence of even numbers from 0 to 8 and range(5,10)
generates a sequence of numbers from 5 to 9. It is commonly used in for loops, to specify the number of iterations to be performed, like for i in range(10)
.
18. How do you create a dictionary in Python?
19. What is the use of the zip() function in Python?
The zip()
function in Python is used to combine two or more iterable objects (such as lists, tuples, or strings) into a single iterable object of tuples. Each tuple in the returned iterable contains an element from each of the input iterables, where the first element of the output tuple is taken from the first element of the first input iterable, the second element of the output tuple is taken from the second element of the second input iterable, and so on.
20. How do you create a lambda function in Python?
In Python, a lambda function is a small, anonymous function that is defined using the lambda
keyword. Lambda functions are also known as “anonymous functions” or “inline functions” because they do not have a name and are defined in a single line. They are useful for short, simple tasks that are not worth defining a separate named function for.
The basic syntax for creating a lambda function is as follows:
lambda arguments: expression
For example, to create a lambda function that squares a number:
square = lambda x: x**2
21. How do you use the map() function in Python?
The map()
function in Python is used to apply a given function to all items of an iterable (such as a list, tuple, or string) and returns a new iterable with the modified items. The function that is used with map()
is called for each item of the input iterable, and its return value is used to create the new iterable.
The basic syntax for using map()
is as follows:
map(function, iterable)
CLICK HERE for more information
22. How do you use the filter() function in Python?
The filter()
function in Python is used to filter the items of an iterable (such as a list, tuple, or string) based on a given function and returns a new iterable with only the items that satisfy the function’s condition. The function that is used with filter()
is called for each item of the input iterable, and its return value is used to determine whether or not the item will be included in the new iterable.
The basic syntax for using filter()
is as follows:
filter(function, iterable)
CLICK HERE for more information
23. What is the use of the reduce() function in Python?
The reduce()
function in Python is a built-in function in the functools
module. It applies a function to a sequence of elements from left to right, so as to reduce the sequence to a single value. The function takes two arguments: the accumulated value and the current value from the sequence. The accumulated value is initialized as the first element of the sequence, and the function is applied to the accumulated value and each subsequent element in the sequence. For example, you could use the reduce()
function and the lambda
function to find the product of all the elements in a list, like this: reduce(lambda x, y: x*y, [1, 2, 3, 4])
. This would return 24, which is the product of all the elements in the list.
CLICK HERE for more information
24. What is the use of the pass in Python?
Pass is a special statement in Python that does nothing. It only works as a dummy statement.
The pass statement in Python is used when a statement is required syntactically but you do not want any command or code to execute. Python Pass
The pass statement is a null operation; nothing happens when it executes. The pass is also useful in places where your code will eventually go, but has not been written yet.
25. What is the use of the break in Python?
Break statement in Python is used when you want to stop/terminate the flow of the program using some condition. Python Break
Assume that you want to exit from for loop & while loop you can use the break
statement to exit a loop in Python
#using break statement in while loop
n = 1
while n < 10:
print(n)
if n == 5:
break
n += 1
26. What is the use of the Continue in Python?
Continue
is also a loop control statement just like the break statement. continue
statement is opposite to that of break statement, instead of terminating the loop, it forces to execute the next iteration of the loop.
As the name suggests the continue statement forces the loop to continue or execute the next iteration. When the continue statement is executed in the loop, the code inside the loop following the continue statement will be skipped and the next iteration of the loop will begin.
for i in range(1, 10):
if i == 5:
continue
print(i)
27. How do you use the sorted() function in Python?
The sorted()
function is used to sort a list of items in ascending or descending order. The basic syntax for using the function is as follows:
sorted(iterable, key=None, reverse=False)
iterable
: The list or other iterable object that you want to sort.key
: An optional argument that specifies a function to be used to extract a comparison key from each list element.reverse
: An optional boolean argument that determines whether the list should be sorted in descending order (True) or ascending order (False).Here is an example of how to use thesorted()
function to sort a list of numbers:
Here is an example of how to use the sorted()
function to sort a list of numbers:
numbers = [3, 1, 4, 2, 5]
sorted_numbers = sorted(numbers)
print(sorted_numbers)
This will output [1, 2, 3, 4, 5]
, which is the list of numbers sorted in ascending order.
28. What is the use of the reversed() function in Python?
The reversed()
function is a built-in function in Python that returns an iterator that produces items of a given iterable in reverse order. It does not modify the original iterable and does not return a new list, it returns a reverse iterator object.
The basic syntax for using the function is:
reversed(iterable)
iterable
: The list, tuple, string, or other iterable object that you want to reverse.
Here is an example of how to use the reversed()
function to reverse a list:
numbers = [1, 2, 3, 4, 5]
reversed_numbers = list(reversed(numbers))
print(reversed_numbers)
This will output [5, 4, 3, 2, 1]
, which is the list of numbers in reverse order.
29.What is the use of the enumerate() function in Python?
The enumerate()
function is a built-in function in Python that allows you to iterate over a list or other iterable object while keeping track of the index of the current item. It returns an iterator that produces tuples containing the index and the corresponding item of the given iterable object.
The basic syntax for using the function is:
enumerate(iterable, start=0)
iterable
: The list, tuple, string, or other iterable object that you want to enumerate.start
: An optional argument that specifies the starting index for the enumeration. By default, it is 0.
Here is an example of how to use the enumerate()
function to iterate over a list and print the index and value of each item:
numbers = [1, 2, 3, 4, 5]
for index, value in enumerate(numbers):
print(index, value)
0 1
1 2
2 3
3 4
4 5
30. How do you use the join() method in Python?
The join()
method is a string method in Python that allows you to join a sequence of strings (e.g. a list or tuple) into a single string. The basic syntax for using the method is:
separator.join(iterable)
separator
: The string that you want to use to separate the items in the iterable.iterable
: The list, tuple, or other iterable object that you want to join into a single string.
Here is an example of how to use the join()
method to join a list of strings:
words = ['cat', 'dog', 'bird']
sentence = ' '.join(words)
print(sentence)
This will output cat dog bird
, which is the concatenation of the words in the tuple separated by a space.
The join()
method can be used to join any iterable object containing strings, the separator can be any string.
It’s worth noting that join()
method must be called on a separator string, not on an iterable object, so you have to specify separator first before using join, and the iterable object is passed as an argument to join method.
31. What is the difference between Python 2 and Python 3?
Python 2 and Python 3 are different versions of the Python programming language. Python 3 introduced several new features and changes, such as improved support for Unicode, improved error handling, and the removal of some deprecated features from Python 2.
32. What is the use of the “self” keyword in Python?
In Python, the “self” keyword is used to refer to the current instance of a class. It is used to access the attributes and methods of a class from within the class itself.
33. How do you check the type of a variable in Python?
In Python, you can check the type of a variable by using the “type()” function. For example: “print(type(x))” will return the type of the variable x.
34. How do you create a for loop in Python?
In Python, you can create a for loop using the “for” keyword, followed by a variable name, the “in” keyword, and an iterable object. For example: “for i in range(10):” creates a for loop that will iterate 10 times.
35. What is the use of the “try-except” block in Python ?
The “try-except” block in Python is used to handle exceptions, which are events that occur during the execution of a program that disrupt the normal flow of instructions. The “try” block contains the code that may raise an exception, and the “except” block contains the code that will be executed if an exception is raised. This allows for the program to gracefully handle errors and continue running instead of crashing.
36. How do you implement a decorator in Python ?
A decorator is a function that takes another function as its argument and extends the behavior of the latter function without modifying its code. Decorators are defined using the “@” symbol, followed by the name of the decorator function.
37.Explain the use of the “with” statement in Python.
The “with” statement is used to wrap the execution of a block of code that uses resources that need to be released after the block has been executed. This ensures that the resources are correctly and automatically cleaned up, even if an exception is raised.
38. How do you implement a generator in Python ?
A generator is a special type of function that returns an iterator, which can be used to iterate over a sequence of values. Generators are defined using the “yield” keyword instead of “return”.
39. Explain the use of the “yield” keyword in Python.
The “yield” keyword is used to define a generator function. When a generator function is called, it returns an iterator object, but it does not execute the function. The function is only executed when the iterator’s “next()” method is called.
40.How do you implement multi-threading in Python ?
Multi-threading in Python is implemented using the “threading” module. To create a new thread, we can create a new instance of the “Thread” class and call its “start()” method.
41. Explain the difference between deep and shallow copying in Python.
Deep copying creates a new object with a new memory address, while shallow copying only creates a new reference to the original object. Shallow copy is faster than deep copy but it might lead to unexpected bugs if not used carefully
42. How do you implement a closure in Python?
A closure is a nested function that has access to the variables in the enclosing scope. Closures are created by defining a nested function within another function and returning the nested function.
43. Explain the use of the “*args” and “**kwargs” in Python function arguments.
The “*args” and “**kwargs” are used to pass a variable number of arguments to a function. “*args” is used to pass a variable number of non-keyword arguments, while “**kwargs” is used to pass a variable number of keyword arguments.
44. Explain the difference between a class variable and an instance variable in Python.
A class variable is a variable that is shared among all instances of a class, while an instance variable is a variable that is specific to a particular instance of a class. Class variables are defined within the class body, while instance variables are defined within the constructor method.
45. How do you implement polymorphism in Python ?
Polymorphism in Python is the ability of a single function or method to work with multiple types of input. This can be achieved through inheritance and method overriding or by using the “duck typing” approach, where the type of an object is determined by its methods and properties rather than its class.
46. Explain the use of the “init” method in Python classes.
The “init” method is a special method in Python classes that is called when an instance of the class is created. It is used to initialize the attributes of the class and is often referred to as the constructor method.
47. Explain the difference between a module and a package in Python.
A module in Python is a single file that contains Python code, while a package is a collection of modules that are organized in a directory hierarchy. Packages allow for better organization of code and help to avoid naming conflicts.
48.Explain the use of the “super()” function in Python classes.
The “super()” function is used to call a method from the parent class in a subclass. It allows for the reuse of the parent class method and customization of the subclass method.
49. What is the difference between a local variable and a global variable in Python?
A local variable is a variable that is defined within a function and can only be accessed within that function. A global variable is a variable that is defined outside of a function and can be accessed from anywhere in the code.
50. How do you implement a singly linked list in Python?
- A singly linked list in Python can be implemented by defining a Node class that contains a data attribute and a next attribute, which points to the next node in the list. The linked list class maintains a reference to the head node and provides methods for adding, removing, and accessing elements in the list.
Intermediate Level ———-
51. What is the difference between shallow copy and deep copy in Python?
A shallow copy creates a new object that points to the same elements as the original object, whereas a deep copy creates a new object with a new memory address and a completely independent copy of the original object.
52. How do you handle exceptions in Python?
Exceptions can be handled in Python using try-except blocks. The code that may raise an exception is placed inside the try block, and the code that handles the exception is placed inside the except block.
53. What is the difference between a tuple and a list in Python?
A list is a mutable sequence of elements, while a tuple is an immutable sequence of elements. This means that once a tuple is created, its elements cannot be modified, while the elements in a list can be modified.
54. What is the purpose of the “with” statement in Python?
The “with” statement is used to wrap the execution of a block of code with methods defined by a context manager. It provides a convenient way to instantiate an object and automatically take care of cleaning up after the block of code has been executed, even in the presence of exceptions.
55. How do you define a class in Python and what is the syntax for creating objects?
A class is defined using the class keyword in Python, followed by the name of the class. The syntax for creating an object from a class is to call the class name as if it were a function. For example:
class MyClass:
def __init__(self):
self.x = 42
obj = MyClass()
56. How do you implement inheritance in Python?
Inheritance is implemented in Python by defining a subclass that inherits from a base class. The subclass can override or extend the methods of the base class by defining its own implementation. The syntax for inheritance in Python is as follows:
class BaseClass:
...
class SubClass(BaseClass):
...
57. What is a decorator in Python, and how do you use it ?
A decorator is a special type of function in Python that is used to modify the behavior of another function. Decorators are applied to a function using the @ symbol, followed by the name of the decorator. For example:
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
58. How can you pass optional and keyword arguments to a function in Python?
Optional arguments can be passed to a function in Python by specifying default values for the arguments in the function definition. Keyword arguments are arguments that are passed to a function by explicitly specifying the argument name, along with its value.
59. What is the difference between range and xrange in Python 2.x ?
The range function in Python 2.x returns a list of numbers, whereas the xrange function returns an xrange object that generates the numbers on the fly. The xrange function is more efficient than the range function because it does not generate the entire list of numbers in memory at once.
60. What is a generator function in Python and how is it used ?
A generator function is a special type of function in Python that generates a sequence of values, one at a time, as it is called. A generator function is defined like a normal function, but instead of using a return statement to return a value, it uses the yield
statement. When the generator function is called, it returns a generator object, which can be used to iterate through the generated values. For example:
def my_generator():
for i in range(3):
yield i
gen = my_generator()
for i in gen:
print(i)
61. How can you make HTTP requests using the requests library in Python?
HTTP requests can be made in Python using the requests library. The library provides a simple interface for making HTTP requests, including GET, POST, and other HTTP methods. For example, here’s how you can make a GET request to retrieve a webpage:
import requests
response = requests.get('https://www.example.com')
if response.status_code == 200:
print(response.text)
62. What are some of the ways to optimize the performance of a Python code? There are several ways to optimize the performance of a Python code, including:
- Use built-in functions and libraries instead of writing custom code
- Avoid using global variables as they are slow to access
- Use the right data structure for the task, such as using a dictionary instead of a list for lookups
- Avoid using loops when possible, and use vectorized operations instead
- Use built-in functions such as map, filter, and reduce to process large amounts of data efficiently
- Use the built-in profiler to find bottlenecks in your code and optimize those areas.
63. How do you handle file input and output operations in Python?
File input and output operations can be performed in Python using the built-in open
function. The open
function takes the file name and the mode (read, write, etc.) as arguments and returns a file object, which can be used to read or write to the file. For example:
# Writing to a file
with open('file.txt', 'w') as file:
file.write('Hello, world!')
# Reading from a file
with open('file.txt', 'r') as file:
content = file.read()
print(content)
64. What is the difference between the sort
and sorted
functions in Python ?
The sort
method is used to sort the elements of a list in-place, while the sorted
function returns a new sorted list. The sort
method modifies the original list and does not return anything, while the sorted
function returns a new list and does not modify the original list. For example:
a = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3]
a.sort() # Sorts the list in-place
print(a) # [1, 1, 2, 3, 3, 4, 5, 5, 6, 9]
b = sorted(a) # Returns a new sorted list
print(b) # [1, 1, 2, 3, 3, 4, 5, 5, 6, 9]
65. How can you make a Python script executable from the command line ?
To make a Python script executable from the command line, you need to add the following line as the first line of your script:
#!/usr/bin/env python3
Then, you need to make the script executable by running the following command:
chmod +x script.py
After that, you can run the script from the command line by typing:
./script.py
66. What is the difference between append
and extend
when working with lists in Python ?
The append
method is used to add a single element to the end of a list, while the extend
method is used to add multiple elements to the end of a list. The append
method takes a single argument, which can be any type of object, while the extend
method takes an iterable object, such as a list, as an argument. For example:
a = [1, 2, 3]
a.append(4) # Adds the integer 4 to the end of the list
print(a) # [1, 2, 3, 4]
a.extend([5, 6, 7]) # Adds the elements of the list [5, 6, 7] to the end of the list
print(a) # [1, 2, 3, 4, 5, 6, 7]
67. How do you perform unit testing in Python, and what is the purpose of the unittest module ?
Unit testing is a software testing technique in which individual units of code, such as functions or methods, are tested in isolation from the rest of the application. In Python, unit testing can be performed using the built-in unittest
module. The unittest
module provides a framework for writing and running tests, and it includes a number of tools and assertions for testing various types of data and conditions. The purpose of the unittest
module is to provide a way to automate the testing process and ensure that changes to the code do not break existing functionality.
Here is an example of a simple unit test using the unittest
module:
import unittest
def add(a, b):
return a + b
class TestAdd(unittest.TestCase):
def test_add(self):
result = add(3, 4)
self.assertEqual(result, 7)
if __name__ == '__main__':
unittest.main()
68. What is the purpose of the __str__
and __repr__
methods in Python classes ?
The __str__
method is used to define a human-readable string representation of an object, which is used by the print
function and str
function. The __repr__
method is used to define a unambiguous string representation of an object, which is used by the repr
function and the interactive interpreter.
The purpose of these methods is to provide a way to represent an object as a string, which makes it easier to understand and debug the object. For example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f'{self.name}, {self.age} years old'
def __repr__(self):
return f'Person({self.name!r}, {self.age!r})'
p = Person('John Doe', 30)
print(p) # John Doe, 30 years old
print(repr(p)) # Person('John Doe', 30)
69. What is the difference between a dynamic and a static programming language, and how does Python fit into these categories ?
A dynamic programming language is a language in which the type of a variable is determined at runtime, while a static programming language is a language in which the type of a variable is determined at compile-time.
Python is a dynamically-typed language, which means that the type of a variable is determined at runtime. This allows for more flexibility and easier prototyping, but it can also make the code more prone to runtime errors.
70. How do you implement a linked list in Python, and what are the benefits and drawbacks of using linked lists compared to arrays ?
A linked list can be implemented in Python using classes and references to objects. Each node in the linked list is represented by an object, which contains a value and a reference to the next node in the list. The first node in the list is referred to as the head, and the last node in the list is referred to as the tail.
The benefits of using linked lists compared to arrays include constant-time insertions and deletions at the head of the list, and the ability to allocate new nodes individually, which can be more efficient in terms of memory usage. The drawbacks of linked lists include slower access times to elements in the middle of the list, and the need to traverse the entire list to find a specific element.
Here is an example implementation of a linked list in Python:
class Node:
def __init__(self, value, next=None):
self.value = value
self.next = next
class LinkedList:
def __init__(self):
self.head = None
def append(self, value):
if not self.head:
self.head = Node(value)
else:
current = self.head
while current.next:
current = current.next
current.next = Node(value)
def search(self, value):
current = self.head
while current:
if current.value == value:
return True
current = current.next
return False
def remove(self, value):
previous = None
current = self.head
while current:
if current.value == value:
if not previous:
self.head = current.next
else:
previous.next = current.next
break
previous = current
current = current.next
linked_list = LinkedList()
linked_list.append(1)
linked_list.append(2)
linked_list.append(3)
print(linked_list.search(2)) # True
linked_list.remove(2)
print(linked_list.search(2)) # False