Here is a list of possible interview questions of React, along with answers to help you prepare for your interview: React Interview Questions
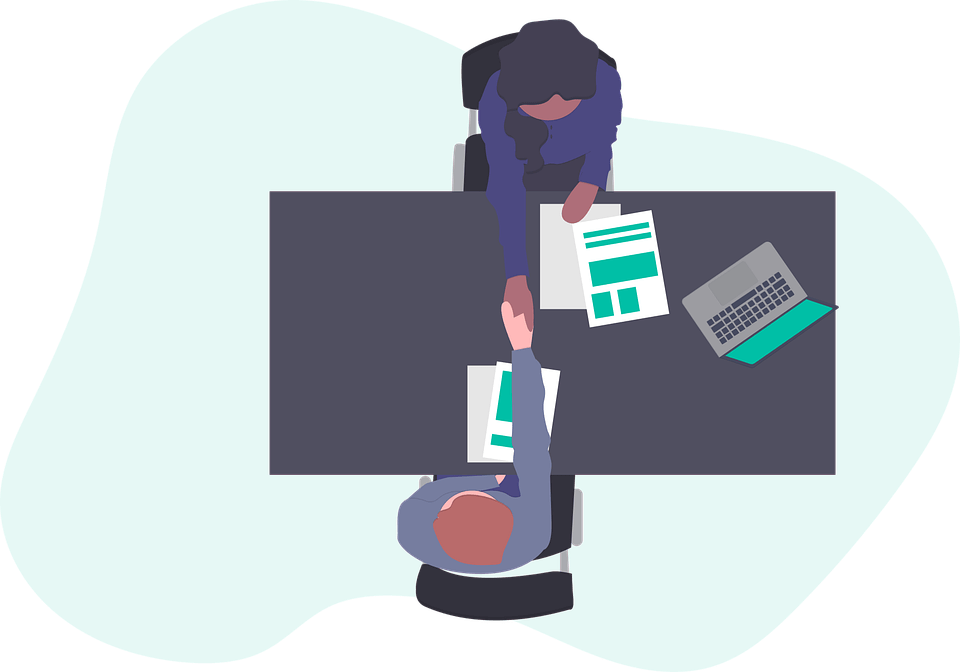
1. What is React and why is it used ?
React is a JavaScript library for building user interfaces. It is used for creating reusable UI components, managing the state of an application, and handling updates efficiently.
2. What are the features of React? React Interview Questions
React is a JavaScript library for building user interfaces. Some of its main features include:
- Components: React is built around the concept of reusable components. Components are independent, self-contained pieces of code that can be easily composed to create complex user interfaces.
- Virtual DOM: React uses a virtual DOM to efficiently update the actual DOM when changes occur. This can greatly improve the performance of a web application, especially when there are many updates happening frequently.
- JSX: React uses JSX, a syntax extension for JavaScript that allows you to write HTML-like elements in your JavaScript code. This makes it easy to define the structure of a component and its children.
- State and Props: React uses a concept of state and props to manage the data flow in an application. Props are used to pass data from a parent component to its children, while state is used to store data that is specific to a component.
- Single-Way Data Flow: React follows a single-way data flow, meaning that data flows from parent components to child components, but not the other way around. This makes it easier to understand and predict the behavior of an application.
- Server-Side Rendering: React allows for server-side rendering, which can improve the performance and SEO of a web application.
- React Native: React can also be used to develop mobile apps using React Native, a framework for building mobile apps using React.
- Support: React has a large and active community of developers, and is widely used in industry, which means that there are a lot of resources and libraries available.
3. How do you create a React app ?
There are several ways to create a React app, but one of the most popular and beginner-friendly methods is to use create-react-app
, a tool that sets up a new React project with a basic file structure and a development server. Here is an example of how to create a new React app using create-react-app
:
1.Install create-react-app globally using npm:
npm install -g create-react-app
2. Create a new project directory and navigate into it:
mkdir my-react-app
cd my-react-app
3. Use create-react-app
to create a new React app:
create-react-app .
4. Once the setup is done, navigate into the project directory:
cd my-react-app
5. Start the development server:
npm start
6.The development server will start and the application will be available at http://localhost:3000. The browser should open automatically.
create-react-app sets up a basic file structure with a src
folder where you can find the main files, such as index.js
and App.js
. It also sets up a development server, webpack, and babel. After the setup is done, you can start building your React app by editing the files in the src
folder and running npm start
to start the development server.
Please note that you need to have Node.js and npm installed on your machine before you can use create-react-app
.
4. Explain about Component in React ?
In React, a component is a reusable piece of code that represents a part of a user interface (UI). It is the building block of a React application, and it allows you to split your UI into small, manageable, and independent parts.
A component can be defined as a JavaScript class or function, and it typically includes a render method that returns a description of what the component should look like when it is rendered.
A component can have its own internal state and behavior, and it can also receive data from its parent component in the form of props. It can also have lifecycle methods which are methods that are called at different stages of the component’s lifecycle, such as when it is first created, updated, or destroyed.
For more info CLICK HERE
5. What is babel in React ?
Babel is a JavaScript transpiler that allows you to use modern JavaScript syntax, such as ES6 and JSX, even if some browsers do not yet support it.
JSX is a syntax extension for JavaScript that allows you to write HTML-like elements in your JavaScript code. It is used by React to describe the structure of a component’s UI. However, most browsers do not natively support JSX, so it needs to be transpiled or converted to regular JavaScript before the browser can understand it.
For more info CLICK HERE
6. Can you explain the Virtual DOM in React ?
In React, the Virtual DOM (Document Object Model) is a lightweight in-memory representation of the actual DOM. It acts as an intermediary between the React components and the actual DOM.
When a component’s state or props change, React first updates the Virtual DOM, which is a lightweight representation of the actual DOM. The updated Virtual DOM is then compared to the previous version using a process called “reconciliation.” This process identifies the specific changes that need to be made to the actual DOM. React then makes those changes to the actual DOM, updating the user interface.
The use of a Virtual DOM improves performance in React because it eliminates the need for costly DOM manipulation operations. When a change occurs, React is able to efficiently update only the parts of the actual DOM that need to be changed, rather than rewriting the entire DOM. Additionally, the Virtual DOM is implemented in JavaScript, so it can be executed in a separate thread, making the updates faster and more responsive.
Overall, the use of the Virtual DOM in React allows for efficient and performant updates to the user interface, improving the overall user experience.
7. What is JSX ?
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like elements in your JavaScript code. It is used by React to describe the structure of a component’s UI.
When you write JSX in a React component, it gets transpiled into regular JavaScript using a tool like Babel.
For more info CLICK HERE
8. What is the significance of the “key” prop in React ?
In React, the “key” prop is used to uniquely identify elements in a list of items. When a list of items is rendered using the map function, React uses the key prop to keep track of which items have been added, removed, or reordered. Without a unique key, React would not be able to determine the identity of the items, and would not be able to efficiently update the list when it changes.
For example, let’s say we have a list of to-do items being rendered in a component, and each item is represented by a component of its own. In this case, we could give each component a key prop that corresponds to the item’s ID. This would allow React to know which component is associated with which item, even if the items are reordered or deleted.
The key prop should be a unique value for each item within the list, and it is recommended to use a unique value that doesn’t change, like an id from a database. It’s also important to note that the key prop is not passed down to the component, so it doesn’t need to be a part of the component’s props. It is only used by React internally to determine the identity of the element.
In summary, the key prop is an important tool for React to maintain the identity of elements in a list and making sure that the proper item being updated, added or removed when there’s an update in the list.
9. What is the difference between state and props in React?
In React, both state and props are used to store data that affects the behavior and rendering of a component. However, they are used in different ways and have some key differences.
- Props (short for “properties”) are used to pass data from a parent component to a child component. They are passed down to a component when it is being initialized and cannot be modified directly by the child component. Props are read-only, and any changes to the data must be done by the parent component, which can then pass the updated data down to the child component through props.
- State, on the other hand, is used to store data that is specific to a component and can change over time. A component’s state can be modified within the component itself by calling the
setState
method, which updates the component’s view with the new state. State is also used to keep track of changes to the component’s internal state, and it will re-render the component when there is a change on the state.
In summary, the main difference between state and props is that props are used to pass data from a parent component to a child component, and state is used to store data that is specific to a component and can change over time. Props are read-only and cannot be modified directly by a child component, while state can be modified by the component that owns it.
10. What is List and Key in React ?
In React, a list is a way to display multiple items or elements of the same type, such as a list of items in a shopping cart or a list of users in a social media application. Lists in React are typically created using the map
function to iterate over an array of data and create a new array of components that represent each item in the list.
A key is a special property that React uses to track and identify each item in a list. It is a unique identifier that React uses to determine which items have been added, removed, or updated when the list is re-rendered.
11. What is the component lifecycle in React?
The component lifecycle in React consists of several methods that are called at different stages of a component’s existence, such as when it is first rendered, when it receives new props, and when it is about to be removed from the DOM.
12. Difference between React and Angular ?
React | Angular |
---|---|
Developed and maintained by Facebook | Developed and maintained by Google |
Uses a virtual DOM to update the actual DOM efficiently | Uses two-way data binding to update the view automatically when the model changes |
Components are written in JavaScript | Components are written in TypeScript, a typed superset of JavaScript |
Uses JSX, a syntax extension for JavaScript that allows you to write HTML-like elements in your JavaScript code | Does not use JSX, instead uses template-based syntax for defining views |
Uses a concept of state and props for data flow | Uses a hierarchy of components and services for data flow |
Has a smaller learning curve for developers familiar with JavaScript | Has a steeper learning curve due to its use of TypeScript and advanced features such as dependency injection |
Please note that both React and Angular are powerful and widely used frameworks, and their capabilities and features are constantly evolving. The above table is a generalization based on current versions and should be used as a general reference.
13. Difference between Virtual and Real DOM ?
Virtual DOM | Real DOM |
---|---|
A JavaScript representation of the actual DOM | The actual structure of the webpage, as interpreted by the browser |
Used to track changes and update the actual DOM efficiently | Directly updates and manipulates the webpage |
Can be used with libraries and frameworks such as React | Accessible through JavaScript using the DOM API |
Only updates the necessary parts of the actual DOM | Every update to the DOM may cause a re-layout and repainting of the entire webpage |
Note that the above table is a generalization, as the implementation of virtual DOMs may vary across different libraries and frameworks. React Interview Questions React Interview Questions React Interview Questions
14. What are Higher-Order Components in React?
A higher-order component (HOC) is a function that takes a component as an argument and returns a new component with additional functionality. HOCs are used to share common functionality among components without repeating code.
15. What is the difference between props and state in React?
Props (short for properties) are used to pass data down from a parent component to its child components. State, on the other hand, is used to store and manage the internal state of a component.
16. What is the Virtual DOM in React?
The Virtual DOM is a lightweight in-memory representation of the actual DOM. React uses the Virtual DOM to efficiently update the UI when the state of an application changes.
17. What is the role of Redux Thunk in React ?
Redux Thunk is a middleware for Redux that allows you to write action creators that return a function instead of an action. This function can then be used to dispatch multiple actions or perform async operations. React Interview Questions React Interview Questions React Interview Questions
18. How does React handle forms?
React handles forms by maintaining the state of the form elements in the component’s state, and by using event handlers to track changes to the form elements. The component can then use the state to render the form, and to handle form submissions.
19. How does React handle performance optimization?
React handles performance optimization by using the virtual DOM to efficiently update the UI when the component’s state or props change. It also uses techniques such as shouldComponentUpdate and React.memo to prevent unnecessary re-renders of components.
20. How does React handle debugging ?
React provides a set of developer tools that can be used to debug a React application. These tools include the React Developer Tools browser extension and the React Native Debugger for mobile development.
21. Can you explain the concept of “higher-order components” in React?
In React, a higher-order component (HOC) is a function that takes a component as an argument and returns a new component that wraps the given component in additional functionality. HOCs are a way to reuse component logic and can be used for tasks such as authentication and performance optimization.
HOCs are used by wrapping a component with another component which then passes props to the wrapped component. It can be defined using following syntax: const EnhancedComponent = higherOrderComponent(WrappedComponent);
HOCs are a powerful tool for reusing component logic and can help keep your code organized and maintainable.
22. What is the purpose of the “setState” method in React?
In React, the setState
method is used to update the state of a component and re-render the component with the new state.
When the setState
method is called, React updates the component’s state and schedules a re-render of the component. The component’s render
method is then called, and the component’s updated state is passed as props to the component’s children.
It’s important to note that React uses a virtual DOM to optimize the re-rendering process. When the setState
method is called, React compares the virtual DOM with the updated virtual DOM, and only makes the necessary changes to the actual DOM. This helps to improve the performance of the application and avoid unnecessary re-renders.
The setState
method accepts an object or a function, the first one updates the state with the passed object and the latter accepts the current state and returns a new state. It is important to keep in mind that setState
is async and multiple calls to setState
may be batched together before React updates the component.
23. How do you handle errors in a React application?
Errors in a React application can be handled using error boundaries, which are components that can catch errors that occur within their child components. You can also use the error-boundary lifecycle method to handle the error and display a fallback UI.
24. How does React handle dynamic forms?
25. Can you explain the concept of “context” in React?
In React, context is a way to pass data through the component tree without having to pass props down manually at every level. It allows you to share values like a current authenticated user and UI theme with all components in the application without passing props through every level of the tree.
To create a context, React provides a createContext
method that accepts a default value as an argument. This value is used when a component is not a descendant of a Provider that provides a value.
For more info Click Here
26. How does React handle styling and CSS?
React handles styling and CSS in several ways:
- Inline styling: React allows you to apply styles directly to individual components using a JavaScript object.
- CSS classes: React allows you to apply CSS classes to components using the className property.
- CSS modules: React allows you to use CSS modules, which are just CSS files that are scoped to a specific component.
- Styled-components: React allows you to use a library called styled-components, which allows you to write CSS styles in JavaScript and apply them directly to your components.
- CSS-in-JS libraries: React allows you to use CSS-in-JS libraries such as Emotion and JSS. These libraries allow you to write CSS styles in JavaScript and apply them directly to your components.
Ultimately, React does not care about how you want to style your components as long as you can apply styles to your components in a way that is consistent with how React handles the DOM.
27. What is the purpose of the “constructor” method in React?
The “constructor” method in React is a special method that is automatically called when a new instance of a class-based component is created. It is typically used to initialize the component’s state and bind any event handlers to the component’s instance.
The constructor method has a few key features:
- The constructor method is the first method that is called when a new instance of a class is created.
- The constructor method is used to initialize the component’s state and set up any necessary data bindings.
- The constructor method is passed the props of the component as its first argument, allowing you to access any props that are passed to the component when it is rendered.
- The constructor method allows you to bind event handlers to the component’s instance, so that the correct context is maintained when the event handlers are called.
- The constructor is an optional method, If you don’t need to initialize the state or bind any event handlers, you don’t need to include a constructor method in your component class.
It’s important to note that with the introduction of React Hooks, it’s more common to use functional components and initialize state using hooks such as useState instead of using class-based components and the constructor method. React Interview Questions React Interview Questions React Interview Questions React Interview Questions React Interview Questions React Interview Questions
28. How does React handle routing?
React handles routing using a library called React Router. React Router is a library that allows you to define a set of routes for your application, and map those routes to specific components that should be displayed when the user navigates to that route.
React Router provides several components that you can use to define and manage your routes, such as:
<BrowserRouter>
: This component is the top-level component for handling client-side routing. It uses the HTML5 history API to keep the UI in sync with the URL.<Route>
: This component is used to define a specific route and map it to a component that should be rendered when the user navigates to that route.<Link>
: This component is used to create links that navigate the user to a specific route when clicked.<Switch>
: This component is used to group together multiple<Route>
components and ensure that only the first matching route is rendered.<Redirect>
: This component is used to redirect the user to a different route.useHistory()
,useLocation()
,useParams()
,useRouteMatch()
: These hooks are used to access the routing-related information in your component, such as the current URL, the URL parameters, and so on.
React Router allows you to easily define and manage client-side routes for your React application, and it is one of the most popular routing libraries for React.
React Interview Questions React Interview Questions React Interview Questions React Interview Questions
29. Can you explain the concept of “portals” in React?
In React, a “portal” is a way to render a component outside of its parent component’s DOM tree. This allows you to place a component in a different part of the DOM, separate from its parent component.
React provides a built-in ReactDOM.createPortal()
method that allows you to create a portal. The method takes two arguments: the first argument is the component you want to render, and the second argument is the DOM node where you want to render the component.
30. What is Redux ?
Redux is a JavaScript library that is commonly used with React for managing the state of a web application. It is based on the principles of the Elm architecture and the concept of a “single source of truth” for application state.
The main features of Redux are: React Interview Questions
- Single Store: Redux uses a single store to hold the entire state of the application. This makes it easy to access and update the state, and to track the changes to the state over time.
- Actions: Redux uses actions to describe the changes that should be made to the state. Actions are plain JavaScript objects that have a
type
property and apayload
property. - Reducers: Redux uses reducers to handle the actions and update the state. Reducers are pure functions that take the current state and an action as inputs, and return a new state as output.
- Middleware: Redux uses middleware to handle asynchronous actions, logging, and other advanced features. Middleware is a way to extend the Redux architecture and add custom functionality.
- DevTools: Redux has a powerful set of developer tools that allow you to track the state changes, inspect the actions, and navigate through the state history.
Redux is often used in combination with React to manage the state of a React application. React components can be connected to the Redux store using the react-redux
library, which provides bindings for React and Redux.
Redux is a powerful tool for managing the state of a web application and it can help to make the code more predictable, maintainable, and testable. However, it can also add complexity to the codebase and should be used only when necessary.
For more info Click Here
31. How do you handle events in React?
In React, events are handled by passing a callback function as a prop to a component. When the event occurs, the callback function is invoked, and it can update the component’s state or call other functions.
32. What is the purpose of Redux in React?
Redux is a library for managing the global state of a React application. It provides a centralized store for all the state in an application, and it allows for predictable changes to the state through the use of actions and reducers. React Interview Questions React Interview Questions React Interview Questions React Interview Questions
33. How does React differ from Angular?
React and Angular are both JavaScript libraries for building web applications, but they have some key differences. React is focused on building reusable UI components, while Angular is a full-featured framework for building web applications. React uses a virtual DOM, while Angular uses change detection to update the UI. React is also unopinionated, whereas Angular provides a more opinionated structure.
34. What is the useContext Hook in React ?
The useContext Hook in React allows a component to access a context, which is a way of passing data down through the component tree without passing props manually at every level.
35. What are the different ways to style components in React?
There are several ways to style components in React, such as using inline styles, CSS classes, CSS modules, and CSS-in-JS libraries like Styled Components.
36. What is the useEffect Hook in React?
The useEffect Hook in React allows a component to synchronize with an external system, such as a web API, or to update the component’s state in response to a change in props or state.
37. What is the useState Hook in React ?
The useState Hook in React allows a functional component to manage its own state. It returns an array with two elements: the current state, and a function to update it.
38. What is the useRef Hook in React ?
The useRef Hook in React allows a component to store a reference to a DOM element, so it can access and manipulate it directly. It also can store a reference to a value that persists across re-renders.
39. What is the useMemo Hook in React ?
The useMemo Hook in React allows a component to cache the result of a costly computation and only re-execute it if certain dependencies change. It returns a memoized value.
40. What is the useCallback Hook in React ?
The useCallback Hook in React allows a component to memoize a callback function, so it only re-creates the function if certain dependencies change. This can improve performance by avoiding unnecessary re-renders.
41. What is the difference between React Router and Reach Router?
React Router and Reach Router are both libraries for routing in React. React Router is the most popular library for routing in React and it’s widely used in the community. Reach Router is a more minimalistic library for routing that’s focused on accessibility. It has a smaller bundle size and some additional accessibility features not present in React Router.
42. What is Server-side rendering (SSR) in React?
Server-side rendering (SSR) is the process of rendering a React application on the server, so that the initial HTML delivered to the client already includes the rendered content. This can improve the performance and SEO of an application.
43. What is the role of Webpack in a React application?
Webpack is a module bundler that is commonly used in React applications to process and bundle JavaScript, CSS, and other assets. It can also be used to transpile code, optimize images, and perform other tasks to prepare an application for production.
44. What is the use of the React Developer Tools browser extension?
The React Developer Tools browser extension is a tool that allows developers to inspect and debug a React application. It allows developers to inspect the component hierarchy, view the component’s props and state, and trace the component’s performance.
45. What is the use of the React DevTools?
React DevTools is a browser extension that allows developers to inspect and debug React components. It provides an interface for inspecting the component hierarchy, viewing component props and state, and tracing component performance.